MAIN CODE:
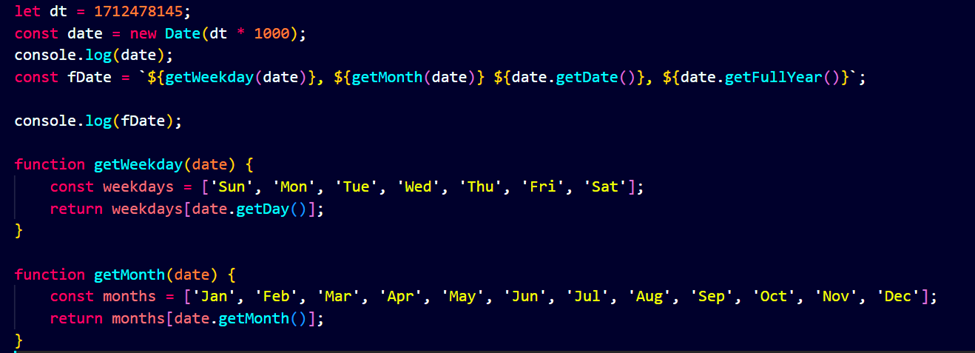
The Unix timestamp 1712478145 represents the number of seconds elapsed since January 1, 1970, 00:00:00 UTC.
CODE BREAKDOWN:
Initially when the date is logged, following output is displayed:
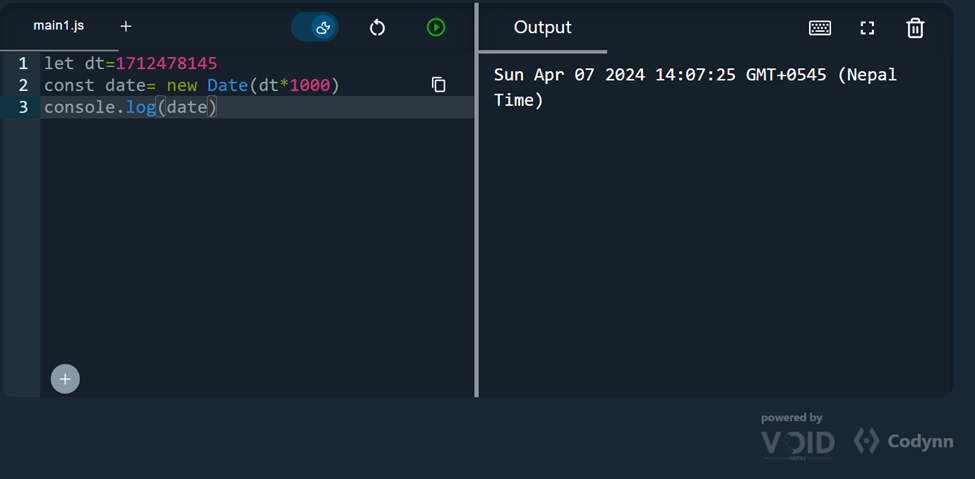
new Date(dt * 1000): This line creates a new Date object named date using the Unix timestamp dt. The Unix timestamp represents the number of seconds since January 1, 1970, 00:00:00 UTC. Multiplying dt by 1000 is necessary because JavaScript’s Date object expects the timestamp to be in milliseconds, not seconds. This line essentially converts the Unix timestamp into milliseconds to create a Date object representing a specific date and time.
In order to break down each of the output data of Day, Month, Date and Year, we add following code.
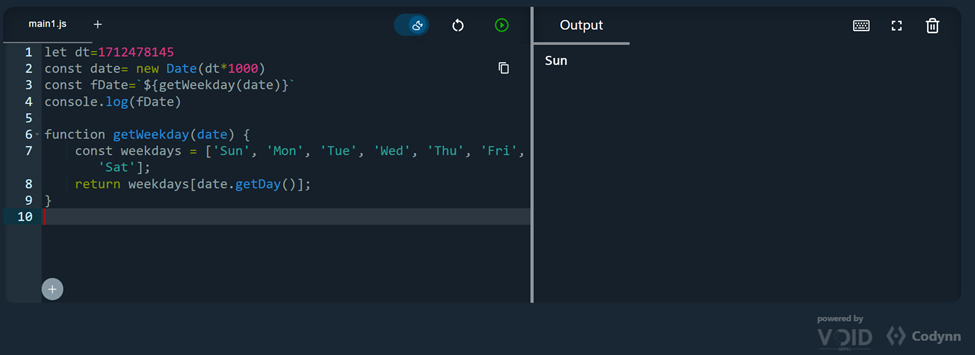
Here we create a function named getWeekday. We pass the date variable as parameter to our newly created function.
date.getDay(): This is a method of the Date object that returns the day of the week (from 0 to 6, where 0 represents Sunday, 1 represents Monday, and so on). In this code, getWeekday function takes a Date object as an argument and returns the weekday abbreviation (e.g., ‘Sun’, ‘Mon’, ‘Tue’, etc.) based on the value returned by date.getDay()
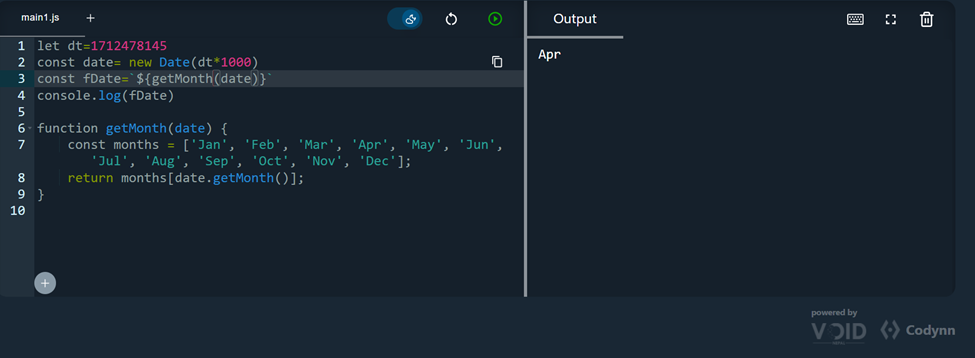
date.getMonth(): This is a method of the Date object that returns the month (from 0 to 11, where 0 represents January, 1 represents February, and so on). In this code, getMonth function takes a Date object as an argument and returns the abbreviated month name (e.g., ‘Jan’, ‘Feb’, ‘Mar’, etc.) based on the value returned by date.getMonth().
Now let’s concatenate both the day and the month into a single string:
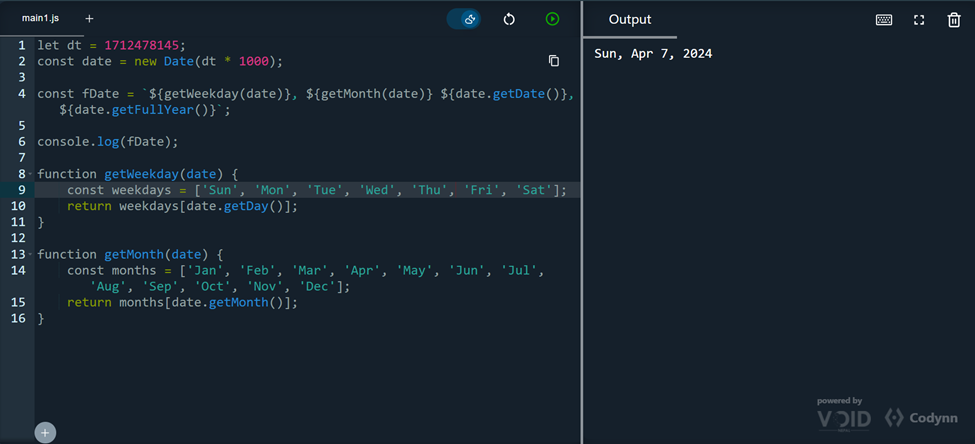
date.getDate(): This is a method of the Date object that returns the day of the month (from 1 to 31) for the specified date. It retrieves the day component of the date represented by the Date object date.
date.getFullYear(): This is another method of the Date object that returns the year (as a four-digit number, such as 2024) for the specified date. It retrieves the year component of the date represented by the Date object date.
SUMMARY:
Initially value of dt is assigned to a variable dt. It then creates a JavaScript Date object named date by multiplying the Unix timestamp by 1000 to convert it into milliseconds, which is the required format for creating a Date object in JavaScript.
- Two helper functions, getWeekday(date) and getMonth(date), are defined to retrieve the abbreviated names of the weekday and month, respectively, from the Date object. These functions use a list of names for weekdays and months to figure out the right names based on the date. The Date object’s getDay() method returns the day of the week as an integer value ranging from 0 (Sunday) to 6 (Saturday). We use this integer value as an index to access the corresponding abbreviated weekday name from an array of weekday names. For example, if date.getDay() returns 0, we access the first element of the weekdays array, which contains ‘Sun’ for Sunday.
- Similarly, the Date object’s getMonth() method returns the month as an integer value ranging from 0 (January) to 11 (December). We use this integer value as an index to access the corresponding abbreviated month name from an array of month names. For example, if date.getMonth() returns 0, we access the first element of the months array, which contains ‘Jan’ for January.
We create a new string that looks like a date, using the day of the week, month name, day of the month, and year. We put these parts together using a special syntax called template literals, which allows us to insert variables directly into the string.