Introduction:
In the fast-changing world of web development, JavaScript has become the go-to language for creating interactive and dynamic websites. To make the development process smoother and improve user experience, developers use JavaScript libraries and frameworks. In this article, we’ll explore three of the most popular ones: React, Vue.js, and Angular. By the end of this blog, you’ll have a clear idea of what each of them offers, their strengths, and when to use them. This will help you pick the best tool for your next web project.
React: The Declarative Powerhouse
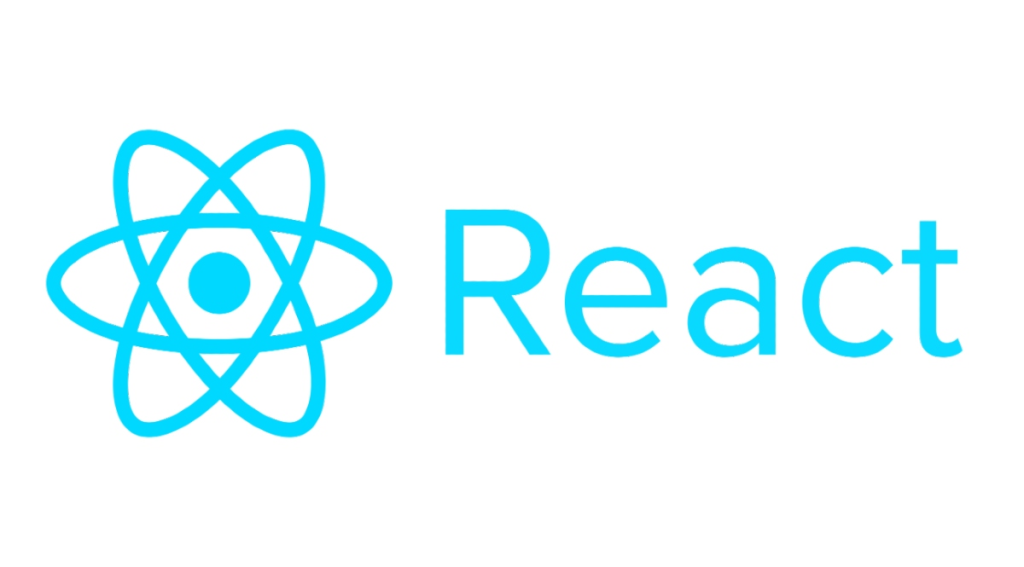
React is a free JavaScript library created by Facebook that is known for its user-friendly method of building interfaces. Instead of directly changing how things appear on the web page, developers describe what the user interface should look like based on the application’s current state. Let’s see an example of a simple React component:
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
const increment = () => setCount(count + 1);
const decrement = () => setCount(count - 1);
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
React is known for its efficient way of updating and showing things on the screen. It does this by using a Virtual DOM, which helps improve performance by reducing the direct manipulation of the actual web page. In React, developers use components, which are like building blocks for their applications. Components help keep the code organized and easy to manage, especially in large projects. React Hooks is a feature that simplifies how developers handle data and actions within components. It makes code cleaner and easier to understand. Also, React is not just for web development; it can be used for mobile app development too. With React Native, developers can write code that works on both iOS and Android devices, saving time and effort.
Vue.js: The Progressive Framework
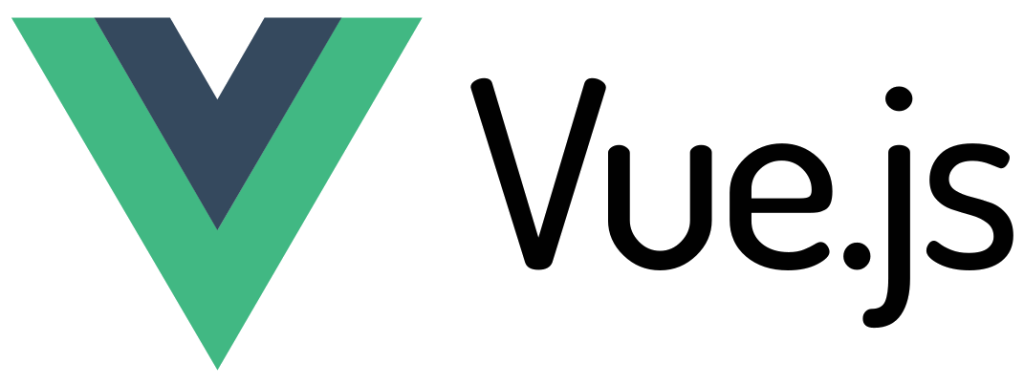
Vue.js is a user-friendly and adaptable JavaScript framework. It’s designed to be accessible and easy to learn. The way you write code in Vue.js looks like a mix of HTML and JavaScript, making it simple for developers to grasp and switch to using Vue.js. Here’s a simple Vue.js component:
<template>
<div>
<p>Count: {{ count }}</p>
<button @click="increment">Increment</button>
<button @click="decrement">Decrement</button>
</div>
</template>
<script>
export default {
data() {
return {
count: 0,
};
},
methods: {
increment() {
this.count++;
},
decrement() {
this.count--;
},
},
};
</script>
Vue.js uses reactivity to keep track of data changes and automatically update the user interface. This means it only updates the parts of the website that need to change, which makes it very efficient. Vue.js also has special attributes called “directives” that start with “v-“. These directives enhance HTML elements, letting developers connect data to the web page, modify the page, or decide when to show elements. To make things even easier, Vue.js includes a helpful tool called Vue CLI. It’s a command-line tool that simplifies various development tasks, like creating projects and optimizing the workflow.
Angular: The Full-fledged Platform
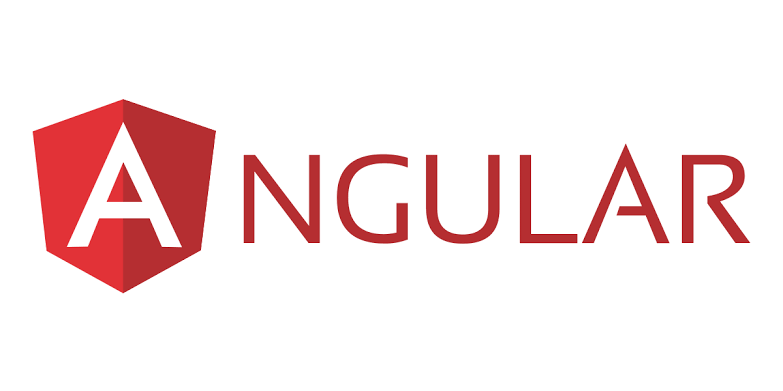
Angular is a powerful JavaScript framework created and maintained by Google. It comes with everything you need to build big applications, including useful tools and best practices. In Angular, applications are organized into modules and components. Modules group related parts of the app together, while components handle specific parts of the user interface with their own logic and templates. One great feature of Angular is that it uses TypeScript, which ensures strong typing and provides helpful tools for checking code errors and making it easier to work with. Angular also relies on something called “dependency injection” to manage how objects are created and used. This promotes code that is modular, reusable, and easy to test. Here’s an example of an Angular component:
import { Component } from '@angular/core';
@Component({
selector: 'app-counter',
template: `
<div>
<p>Count: {{ count }}</p>
<button (click)="increment()">Increment</button>
<button (click)="decrement()">Decrement</button>
</div>
`,
})
export class CounterComponent {
count = 0;
increment() {
this.count++;
}
decrement() {
this.count--;
}
}
The Angular CLI is a helpful command-line tool that makes development tasks easier for developers. It allows them to concentrate on writing code and creating features without getting bogged down by other complex processes.
A Comparative Analysis: Strengths and Use Cases
When it comes to performance, both React and Vue.js are known for being lightweight and efficient. This makes them great choices for smaller to medium-sized projects. Studies and tests show that React’s Virtual DOM and Vue.js’s reactivity system help them deliver fast and smooth user experiences, even when dealing with complex user interfaces.
Real-life Examples
- React: Companies like Facebook, Instagram, Airbnb, and WhatsApp use React to create quick and interactive user interfaces.
- Vue.js: Vue.js is the choice of companies like Alibaba, Xiaomi, and Grammarly for building scalable and high-performing web applications.
- Angular: Big players like Google, Microsoft, and IBM prefer Angular for their enterprise-level applications and internal tools.
Community and Ecosystem
- React: React has a big community with lots of extra libraries and tools available, such as Redux for managing the application’s state and Next.js for server-side rendering.
- Vue.js: Vue.js has a growing and lively community. It also offers popular packages like Vuex for state management and Nuxt.js for server-side rendering and creating static websites.
- Angular: Angular’s community is strong and has a wide range of tools. It includes NgRx for state management and Angular Universal for server-side rendering.
Case Studies
- Airbnb’s switch to React made their code easier to maintain and improved the user experience, resulting in higher conversion rates.
- Grammarly’s move to Vue.js made their website load faster and reduced the time it takes for users to interact with it, leading to increased user engagement.
Tips for Choosing
- For small to medium-sized projects with a focus on high performance and ease of development, consider React or Vue.js.
- For large-scale enterprise applications requiring strong typing, dependency injection, and a comprehensive toolset, Angular may be the preferred choice.
- Assess your team’s expertise and familiarity with each technology before making a decision.
The Future of JavaScript Libraries and Frameworks
The future of web development will be influenced by new trends and exciting innovations. One significant change is the rise of web components, which will become a standard way of building components for websites. State management solutions like Redux for React, Vuex for Vue.js, and NgRx for Angular will keep improving, making it easier to handle complex application states. Progressive Web Apps (PWAs) are becoming more popular, and frameworks like React, Vue.js, and Angular will adapt to include features and optimizations specific to PWAs. JavaScript libraries like React Native will continue to be essential as they help bridge the gap between web and mobile development. This way, developers can create mobile apps that feel native using familiar web technologies.
Conclusion
JavaScript libraries and frameworks have completely transformed web development, giving developers powerful tools to build dynamic, interactive, and efficient web applications. Among the giants in this field are React, Vue.js, and Angular, each catering to different needs and preferences. Understanding their strengths and best uses empowers developers to make smart decisions and embark on exciting web development projects.
As the world of web development keeps evolving, it’s crucial to stay up-to-date with the latest trends and innovations. This will enable developers to create cutting-edge applications that truly enhance the digital experience. By harnessing the capabilities of React, Vue.js, and Angular, developers can craft outstanding web applications that redefine user experiences and drive the web forward.