41. Create a flowchart and an algorithm to display simple interest
Algorithm:
Step 1: Start
Step 2: input Principal, Time, Rate. (P, T, R)
Step 3: I = P * T *R / 100
Step 4: Print “ Simple Interest “ I
Step 5: Stop/End
Flowchart:
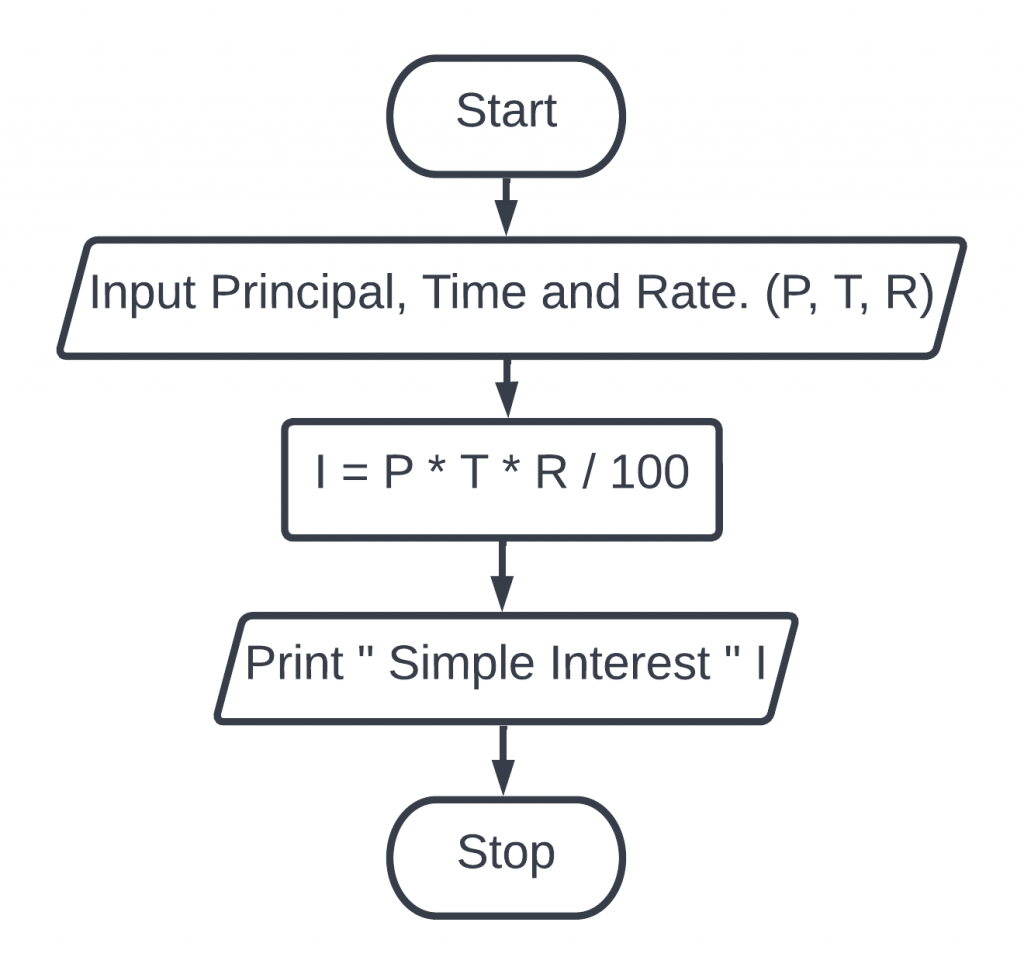
42. Create a flowchart and an algorithm to input number as paisa and convert into rupees only.
Algorithm:
Step 1: Start
Step 2: Input Paisa. (P)
Step 3: RS = P / 100
Step 4: Print “ Rupees value = “ RS
Step 5: Stop/End
Flowchart:
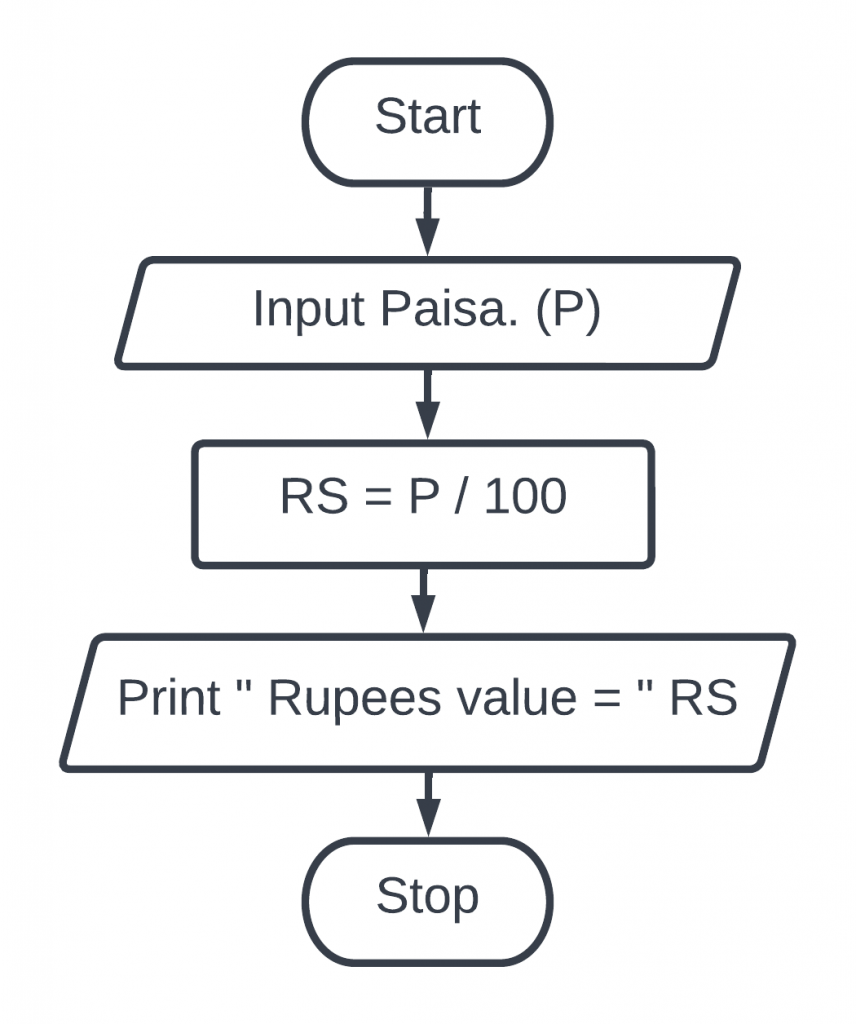
43. Create a flowchart and an algorithm to input selling price and cost price calculate profit or loss percentage.
Algorithm:
Step 1: Start
Step 2: Input cost price and selling price (cp, sp)
Step 3: If cp > sp Then
GoTo Step 4
Else if cp < sp GoTo Step 5
Else GoTo Step 6
Step 4: LP = ((cp – sp) / cp) * 100
GoTo Step 7
Step 5: PP = ((sp – cp) / cp) * 100
GoTo Step 8
Step 6: Print Neither Profit or Loss
GoTo Step 9
Step 7:Print Loss Percentage is : LP
GoTo Step 9
Step 8: Print Profit Percentage is : PP
Step 9: Stop/End
Flowchart:
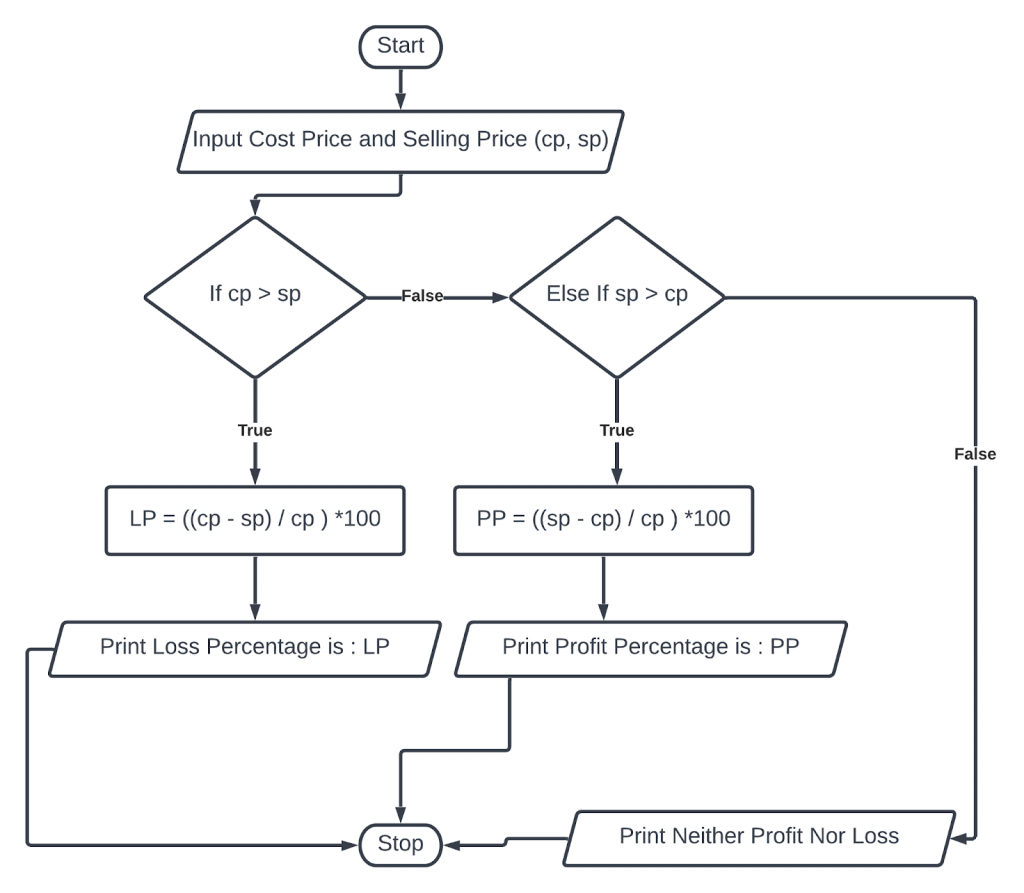
44. Create a flowchart and an algorithm to input the day and display whether the day is Saturday or not.
Algorithm
Step 1: Start
Step 2: Input the today’s day. d
Step 3: If d = “SATURDAY”
GoTo Step 4
Else GoTo Step 5
Step 4: Print “ Today is Saturday “
GoTo Step 6
Step 5: Print “ Today is Not Saturday ”
Step 6: Stop/End
Flowchart:
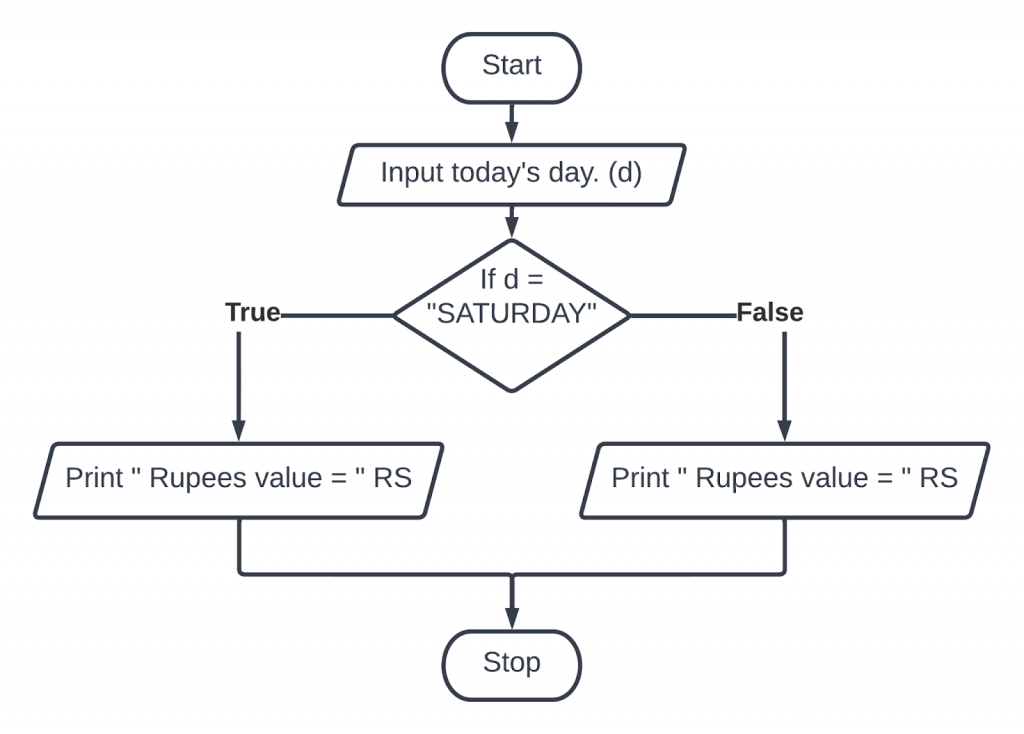
45. Create a flowchart and an algorithm to ask quantity of pen, copy and pencil and their rate and find out the total amount.
Algorithm:
Step 1: Start
Step 2: Input Quantity and Rate Pen, Copy and Pencil. (QP, RP, QC, RC, QPC, RPC)
Step 3: TA = ( QP * RP ) + ( QC * RC ) + ( QPC * RPC )
Step 5: Print “ Total Amount = “ TA
Step 6: Stop/End
Flowchart:
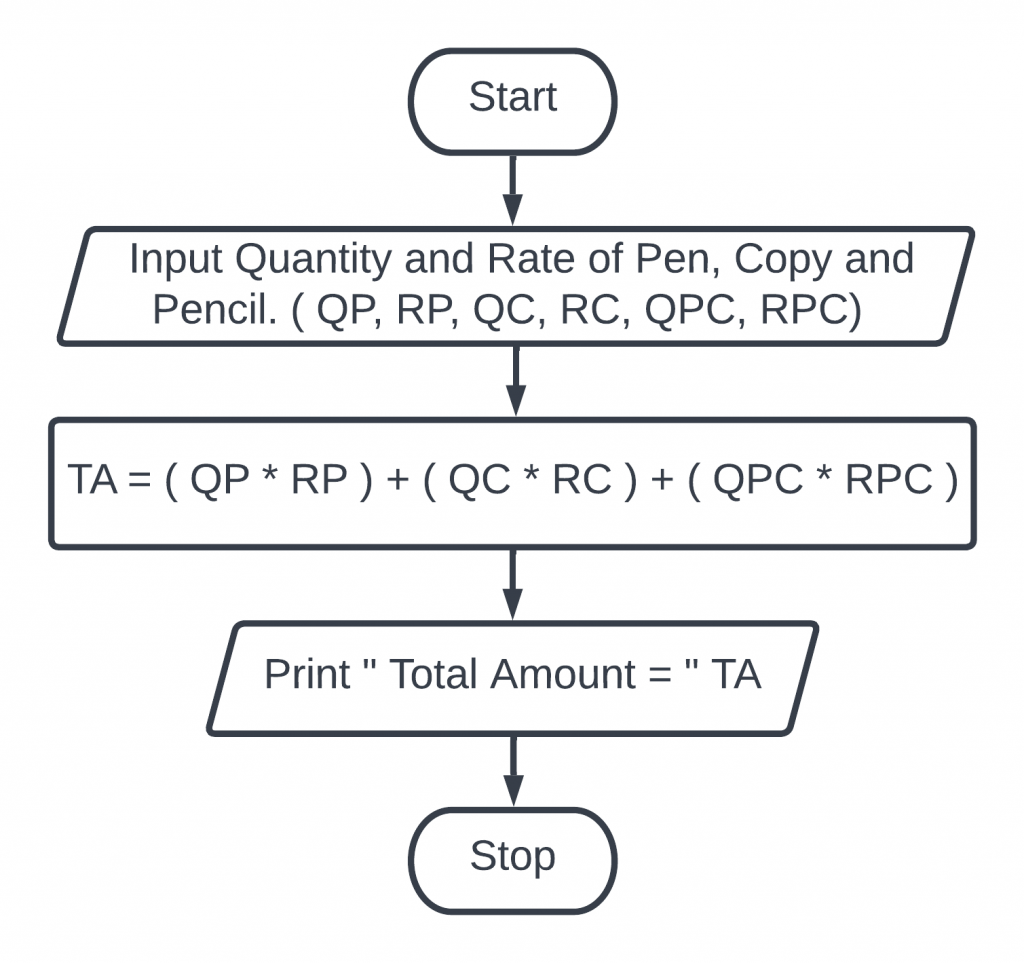
46. Create a flowchart and an algorithm to convert decimal number to octal number.
Algorithm:
Step 1: Start
Step 2: input Decimal Number(D)
Step 3: While D < > 0
If True GoTo Step 4
Else GoTo Step 7
Step 4: R = D MOD 8
Step 5: S = str(R) + S
Step 6: D / 8
GoTo Step 3
Step 7: Print “Octal Equivalent Number is” S
Step 8: Stop/End
Flowchart:
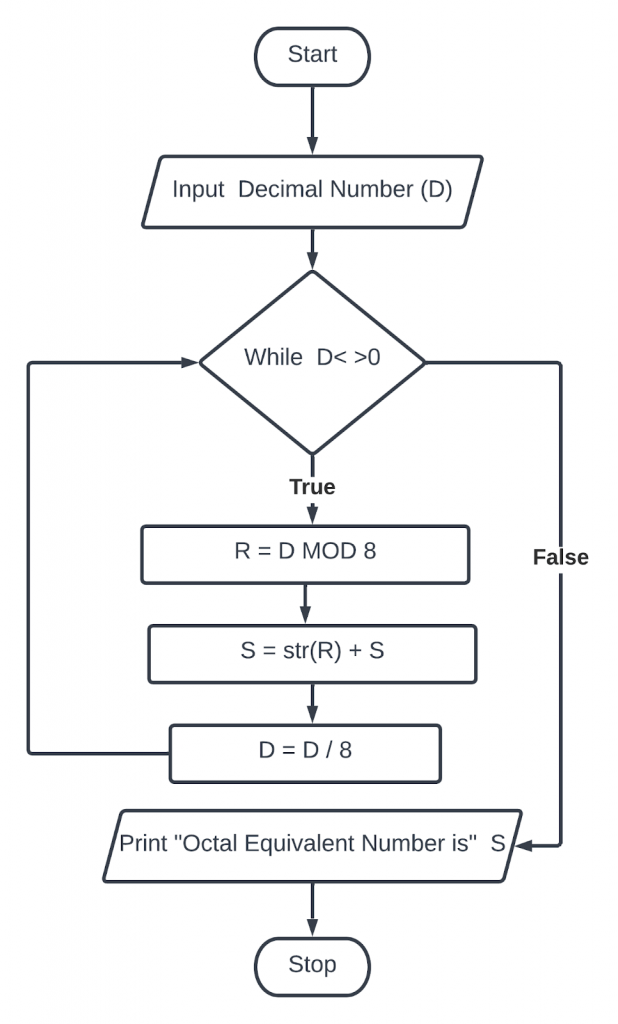
47. Create a flowchart and an algorithm to ask a number and find the sum of cube numbers.
Algorithm:
Step 1: Start
Step 2: Input Any Number. ( N )
Step 3: S = 0
Step 4: While N<>0
If True GoTo Step 5
Else GoTo Step 9
Step 5: R = N MOD 10
Step 6: If R MOD 2 = 1
If True GoTo Step 7
Else GoTo Step 8
Step 7: S = S + R ^ 3
Step 8: N = N \ 10
GoTo Step 4
Step 9: Print “ Sum Of Cube Of Odd Digits “ S
Step 5: Stop/End
Flowchart:
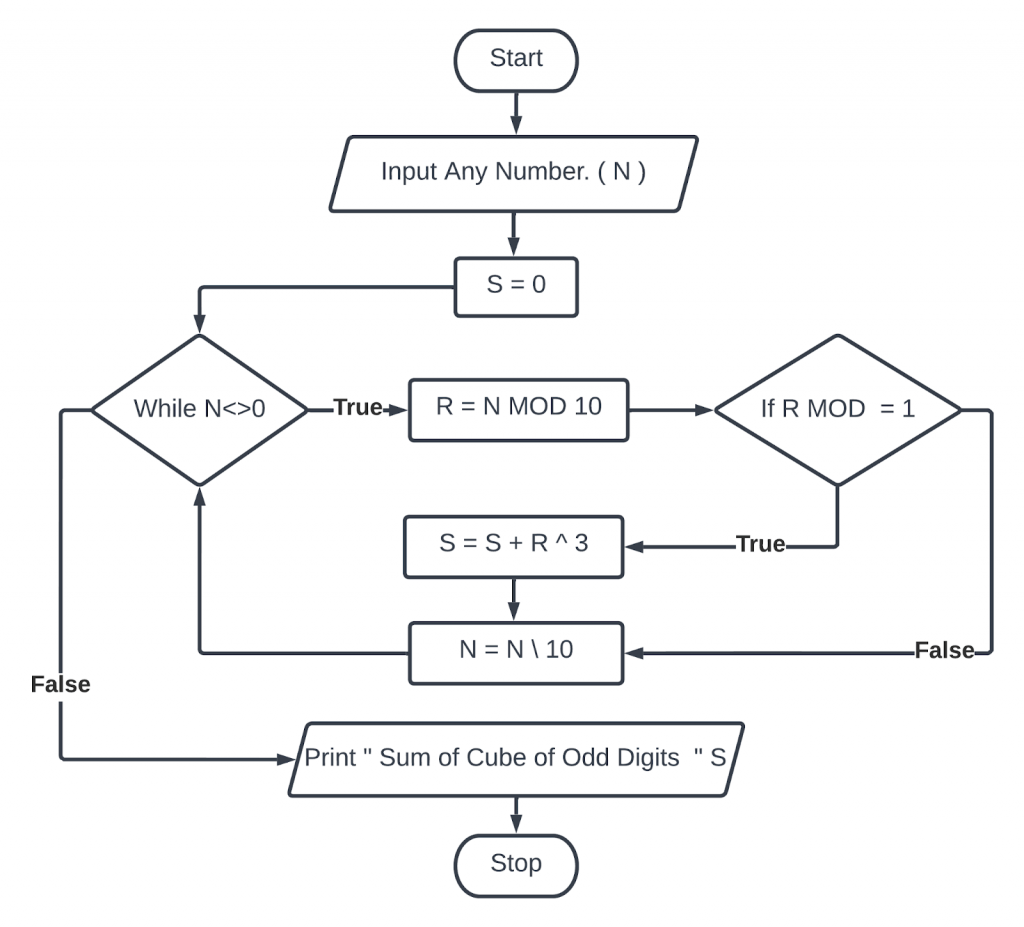
48.Create a flowchart and an algorithm to print 1 10 101 1010 10101
Algorithm:
Step 1: Start
Step 2: For i = 1; i<=5; i =i + 1
For j = 1 ; i<=1; j = j +1
If true GoTo Step 3
Else GoTo Step 4
Step 3: Print j MOD 2
GoTo Step 4
Step 4: Stop/End
Flowchart:
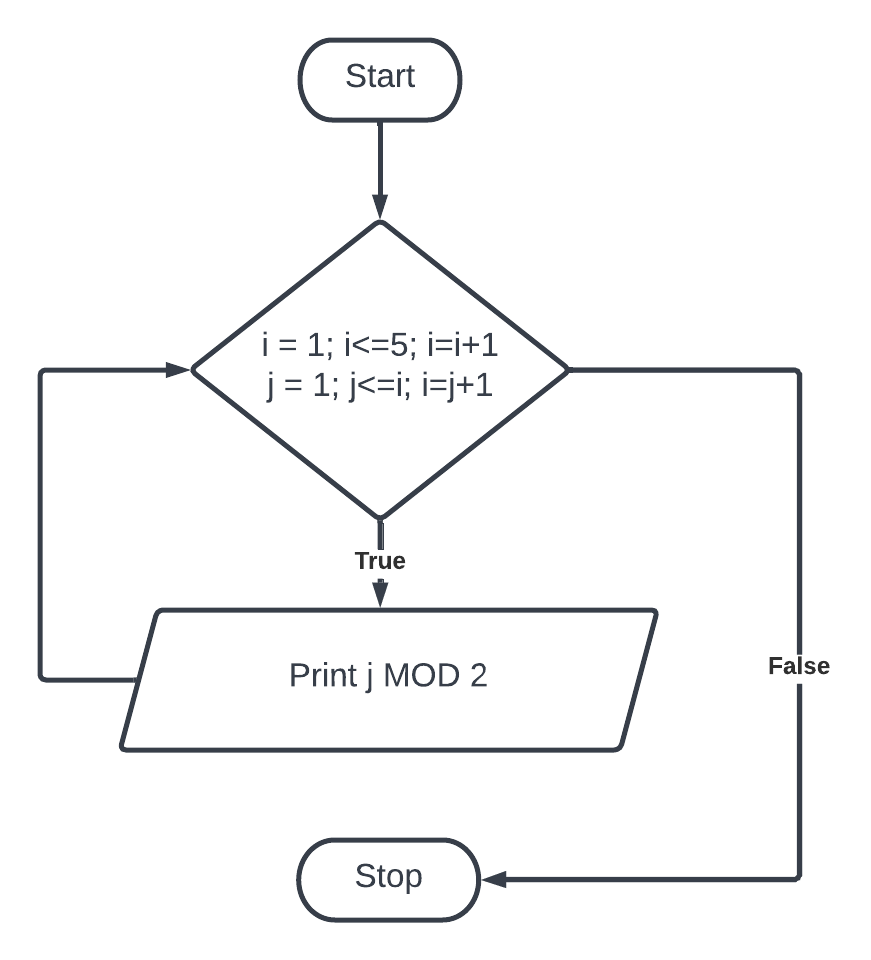
49. Create a flowchart and an algorithm to find the sum of all natural numbers from 1 to 100.
Algorithm :
Step 1: Start
Step 2: For I = 1 To 100
If True GoTo Step 3
Else GoTo Step 4
Step 3: S = S + I
GoTo Step 2
Step 4: Print “ Sum of All Natural Number from 1 to 100 “ S
Step 5: Stop/End
Flowchart:
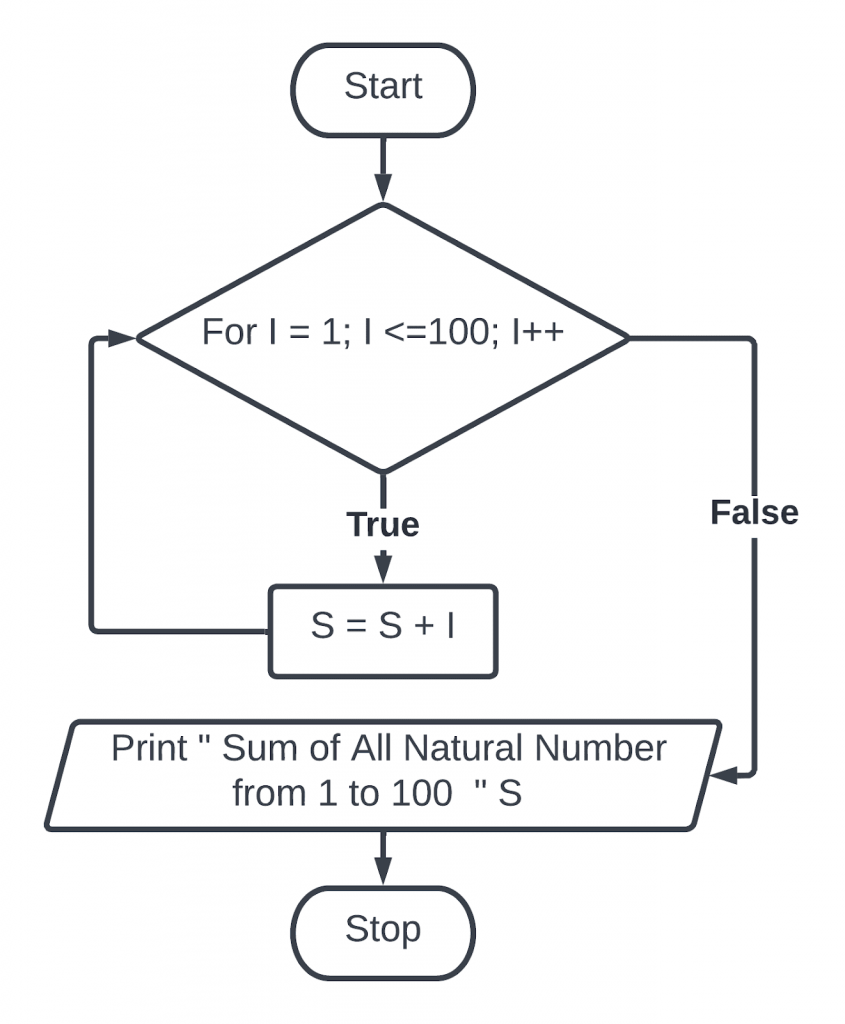
50. Create a flowchart and an algorithm to generate the following series 1 121 12321 1234321 123454321.
Algorithm:
Step 1: Start
Step 2: A = 1
Step 3: for i = 1; i <= 5; i = i + 1
If True GoTo Step 4
Else GoTo Step 6
Step 4: Print A ^ 2
Step 5: A = A * 10 + 1
Step 6: Stop/End
Flowchart:
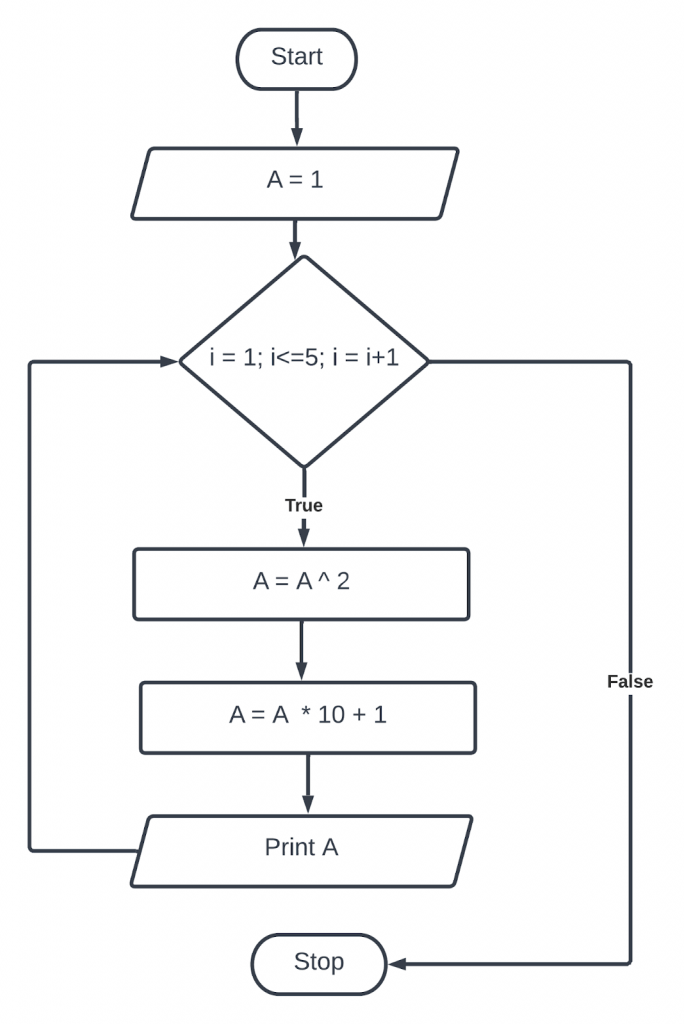