Variable:
A variable is a symbolic representation which refers to or points to an object. Also, it is defined as a container for storing the values. The variable name can contain letters from (A-Z or a-z), digits from (0-9), and an underscore _ but the name must start from either letters or underscore characters. Both the uppercase or lowercase character is acceptable for variable names. But, the reserved keywords which were designed for functionality cannot be declared as variables. In python language, there are altogether 33 reserved keywords. The list of reserved keywords can be viewed by typing help(“keywords”) into the interpreter.
Let’s be clear by the program.
Program:
# This program adds two numbers
x = 4
y = 6
# Add numbers
output = x + y
# printing result
print('The sum of x and y is',output)
Output:
The sum of x and y is 10
In the above program, the x and y are the variables which reserved memory location to store values. It is a program to add two numbers where the two numbers were added and stored in the output variable. After that, the output is printed.
Another example:
Program:
x = 5
y = 4
x = 3 # assigning a new value
z = x + y
print(z)
Output:
7
In the above addition program, x and y are assigned as 5 and 4 respectively. Later, the new value of x is assigned as 3. The actual value of x will be 3 as the program takes the latest assigned value.
Constants:
A constant is a kind of variable that has a fixed value and can’t be changed. The difference between variable and constant is that constant has a fixed value where variable value can be changed during the program’s execution. In most cases of python, constants are declared and allocated in a module. The module is a new file which contains variables, functions, etc. and is imported to the main file in required situations.
Program:
Inside a constant.py file, the constants were declared.
PI = 3.14
GRAVITY = 9.8
Inside a main.py the declared constants were imported.
Program:
import constant
print(constant.PI)
print(constant.GRAVITY)
Output:
3.14
9.8
As we know, the values of PI and GRAVITY were constant and will remain the same. So, the values were declared in a file named as constant and imported to the main file by using the import module.
Literals:
Literals is the raw data given into the variable and constant. There are various types of literals in python such as numeric literals, string literals, boolean literals, special literals, and literal collections.
i. Numeric literals:
The numbers assigned to the variables or constants are numeric literals. Eg; number = 20, the number is variable and the 20 is numeric literals.
ii. String literals:
The set of characters enclosed inside a single or double quote and assigned to the variable or constants is known as string literals. Eg; name = “Subid”, the name is a variable and the “Subid” is a string literal.
iii. Boolean literals:
There are two boolean literals in python which are true or false. The true represents value 1 and false represents value 0. Eg; value = True + 3, the output will be 4. So, the True is a boolean literals.
iv. Literals Collection:
The literals collection contains four different literals which were list literals, tuple literals, dictionary literals, and a set literals.
v. Special Literals:
Python language has one special literals as None which is used to define a null variable. Eg; Value = None, the value is a variable and None is a special literals.
Character Set:
Character is the set of valid characters that are acceptable and recognized by the language. It includes letters, digits and special symbols.
Letters – A to Z and a to z
Digits – 0 to 9
Special Symbols – + – * / etc. and whitespaces
ASCII and Unicode character
Operator:
Operators are the symbols which perform operations on values and variables. Python splits the operators into arithmetic operators, assignment operators, comparison operators, logical operators, identity operators, membership operators, and bitwise operators.
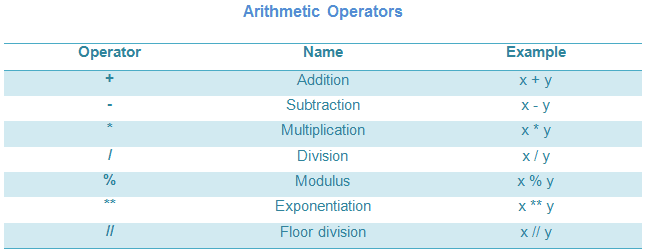
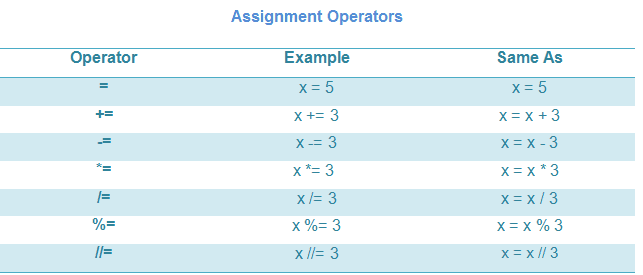
Program:
# This program multiply two numbers
x = 5
y = 7
# Add numbers
output = x * y
# printing result
print('The multiplication of x and y is',output)
Output:
The multiplication of x and y is 35
From the above program, the multiplication (*) is the arithmetic operator which plays a role between the variables to calculate an output.
List:
A list is a set of elements enclosed inside the square brackets [ ] and separated by the comma. It is one of the versatile data types because it allows you to work with multiple elements at once. Normally, we used to create a shopping list, to-do list, etc. for memorizing in our daily life. The items on the list are changeable, ordered, and can have duplicate values. As the same, the python list has similar attributes as it is changeable, ordered, and can have duplicate values.
Program:
# lists
list = ['4','6','7','8','9']
# first item
print(list[1])
# fourth item
print(list[4])
Output:
6
9
From the above program, we print the element of the list. The index starts from 0. So, the first element is 6 and the fourth element is 9.
Tuple:
Tuple is a data type where the elements were inside the parentheses () and separated by the comma. Tuples can have items of different types such as integer, float, string, etc. The question may arise as why to use tuple instead of list? It is because tuple is more memory efficient then the list. Also, it is a slight advantage in terms of time-efficient. A tuple can contain elements of different data types but it is immutable in nature. Immutable objects are those objects whose internal state can’t get changed.
Example for immutable objects:
Program:
values = ("a", "b", "c")
values[2] = "d"
print(values)
Output:
TypeError: 'tuple' object does not support item assignment
In the above program, it throws the error as the object does not support item assignment because tuple is immutable in nature.
Another example:
Program:
test = ('a','b')
# first item
print(test[1])
print(test)
Output:
b
('a', 'b')
From the above program, we print the 1 index value of the tuple and the test. Also, the tuple index starts from 0. So, the 1 index value is ‘b’.
Sets:
A set is an unordered collection of items enclosed inside curly braces {} separated by the comma. Sets can have items of different types such as integer, float, string, etc. Both the list and tuple are ordered collections of items whereas a set is an unordered collection of data type which is iterable, mutable, and without duplicate elements. The items of sets cannot be changed or replaced whereas items of list can be changed or replaced.
Program:
# set of integers
test = {1, 2, 3}
print(test)
Output:
{1, 2, 3}
From the above program, we print the test sets. The items of the sets are enclosed by the {} curly braces and separated by comma.
Dictionaries:
Dictionary is the unordered collection of the items which includes the pair of key and value. It is enclosed in curly braces {}, separated by comma and in the structure of (key: value). Dictionaries contain key-value pairs whereas key is unique and immutable. The values of the dictionary are changeable but duplicate values were not allowed.
Program:
# dictionary
dict = {1: 'Python', 2: 'Java'}
print(dict[1])
print(dict)
Output:
Python
{1: 'Python', 2: 'Java'}
From the above program, we print the 1 key value which is python and print the dict dictionaries.