30. Create a flowchart and an algorithm to display the volume of the hemisphere.
Algorithm:
Step 1: Start
Step 2: Input Radius. ( R )
Step 3: V = ( 2 / 3 ) * 3.14 * R ^ 3
Step 4: Print “ Volume of Hemisphere” V
Step 5: Stop/End
Flowchart:

31. Create a flowchart and an algorithm to display total surface area and volume of cube.
Algorithm:
Step 1: Start
Step 2: Input Length. (L)
Step 3: A = 6 * L ^ 2
Step 4: V = L ^ 3
Step 5: Print “ Total Surface Area of Cube ” A
Step 6: Print “ Volume of Cube ” V
Step 7: Stop/End
Flowchart:
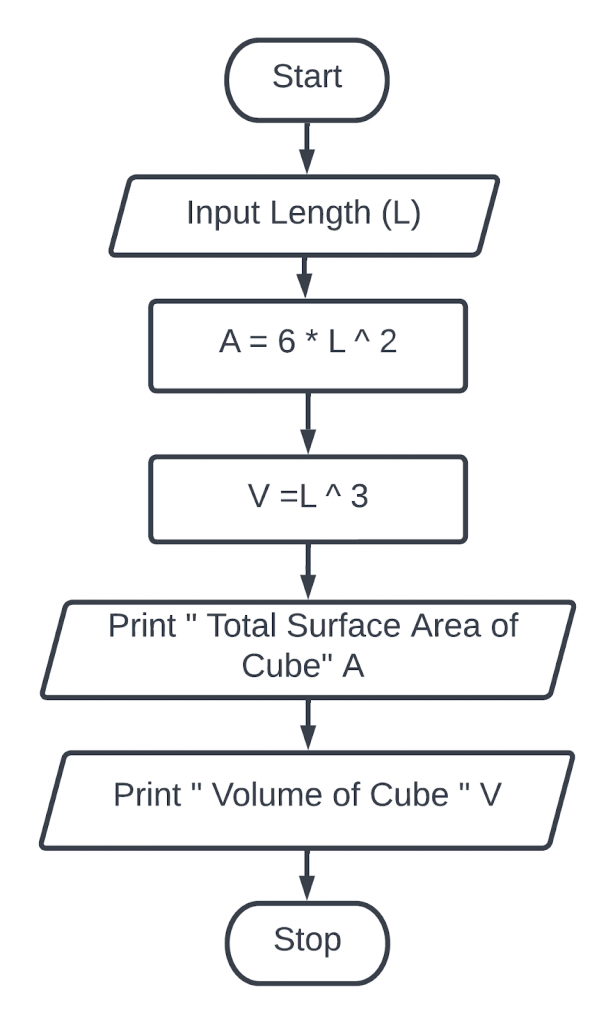
32 . Create a flowchart and an algorithm to the area of the triangle.
Algorithm:
Step 1: Start
Step 2: Input Breadth and Height. (B, H)
Step 3: A = 1/2 * ( B * H )
Step 4: Print “Area of triangle“ A
Step 5: Stop/End
Flowchart:
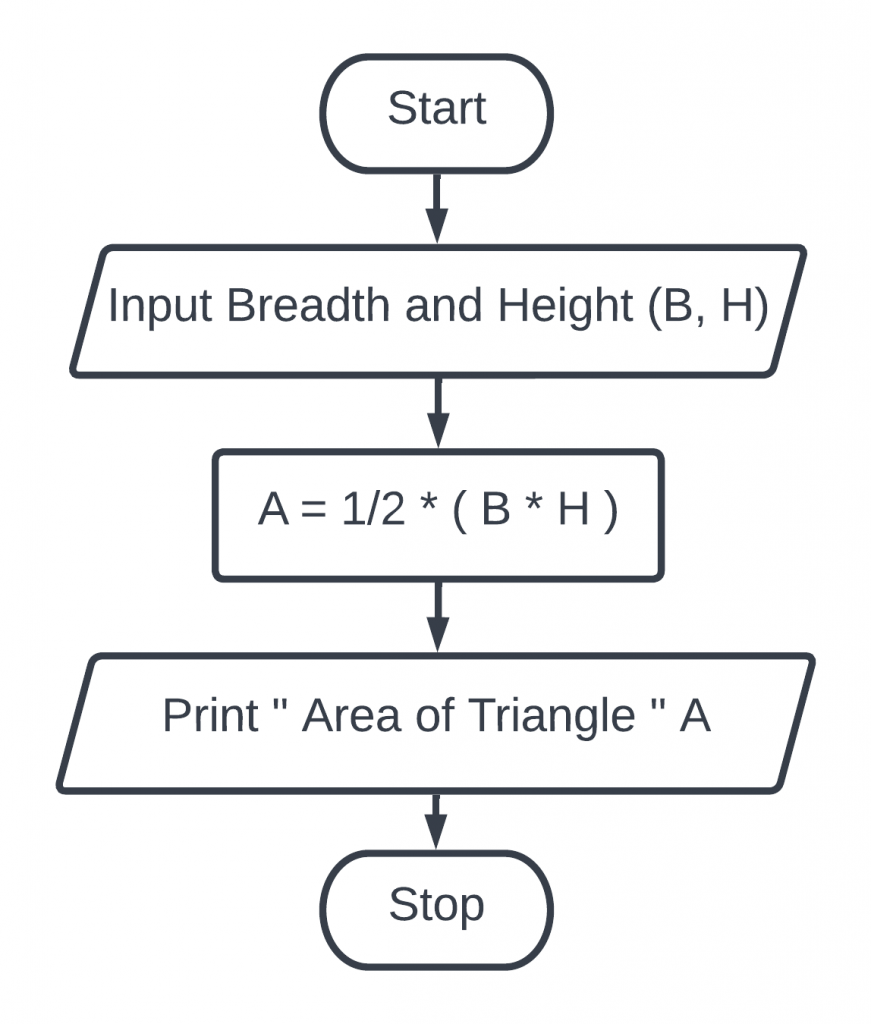
33. Create a flowchart and an algorithm to calculate the volume of a cube.
Algorithm:
Step 1: Start
Step 2: Input Length. (L)
Step 3: V = L ^ 3
Step 4: Print “ Volume of Cube “ V
Step 5: Stop/End
Flowchart:
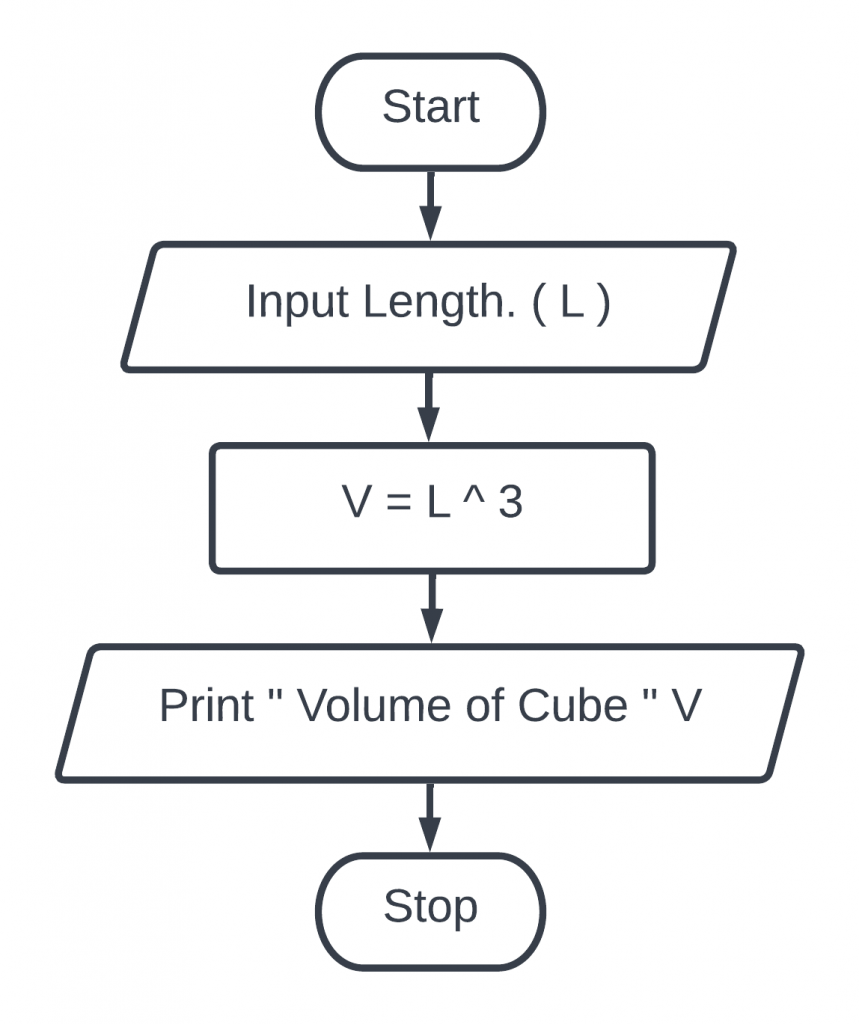
34.Create a flowchart and an algorithm to display the perimeter of the square.
Algorithm:
Step 1: Start
Step 2: Input length of square(l)
Step 3: p = 4 * l
Step 4: Print p
Step 5: Stop/End
Flowchart:
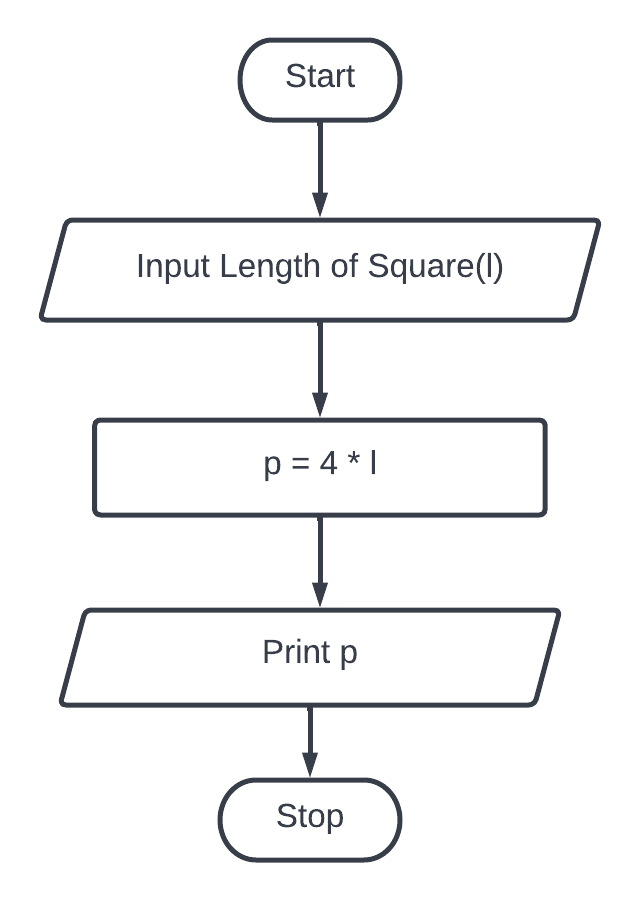
35. Create a flowchart and an algorithm to display the area of parallelogram.
Algorithm:
Step 1: Start
Step 2: Input breadth and height of parallelogram(b, h)
Step 3: A = b * h
Step 4: Print Area is: A
Step 5: Stop/End
Flowchart:
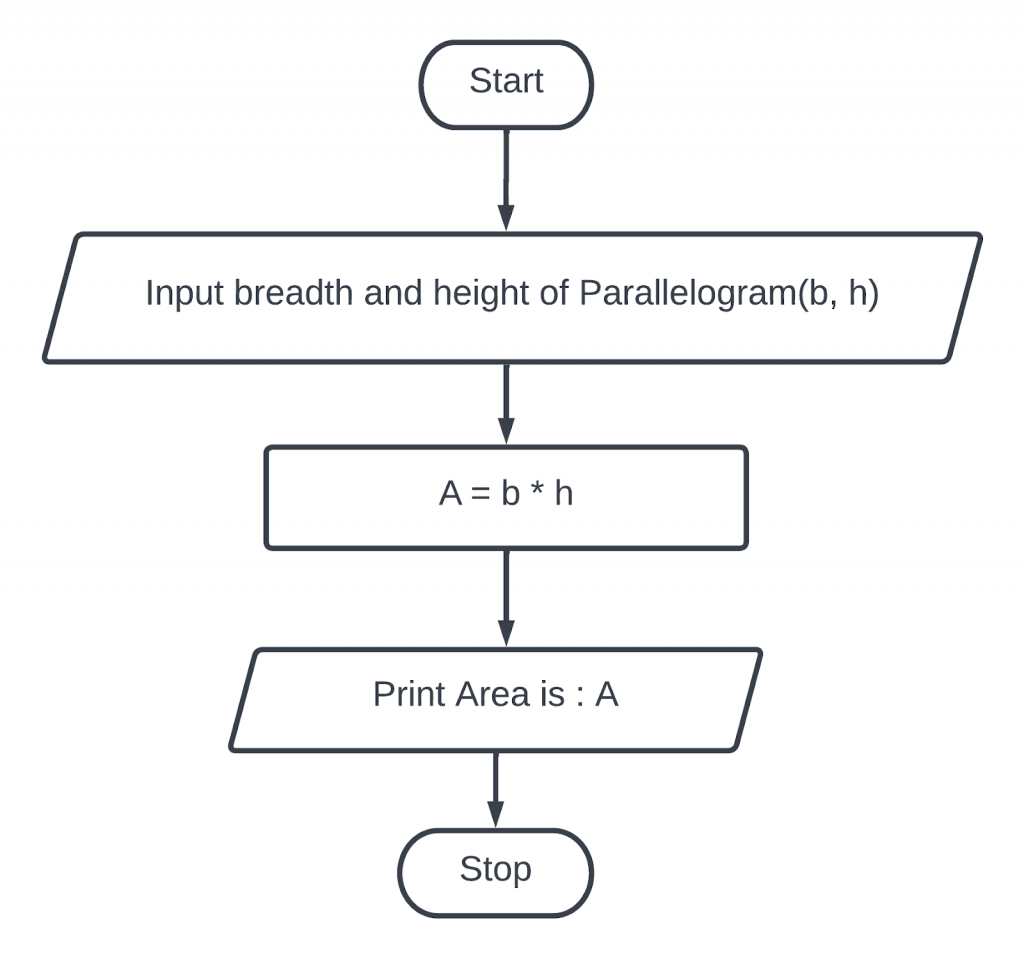
36.Create a flowchart and an algorithm to display the volume of the cuboid/box.
Algorithm:
Step 1: Start
Step 2: input Length, Breadth and Height. (L, B, H)
Step 3: V = L * B * H
Step 4: Print “ Volume of Cuboid “ V
Step 5: Stop/End
Flowchart:
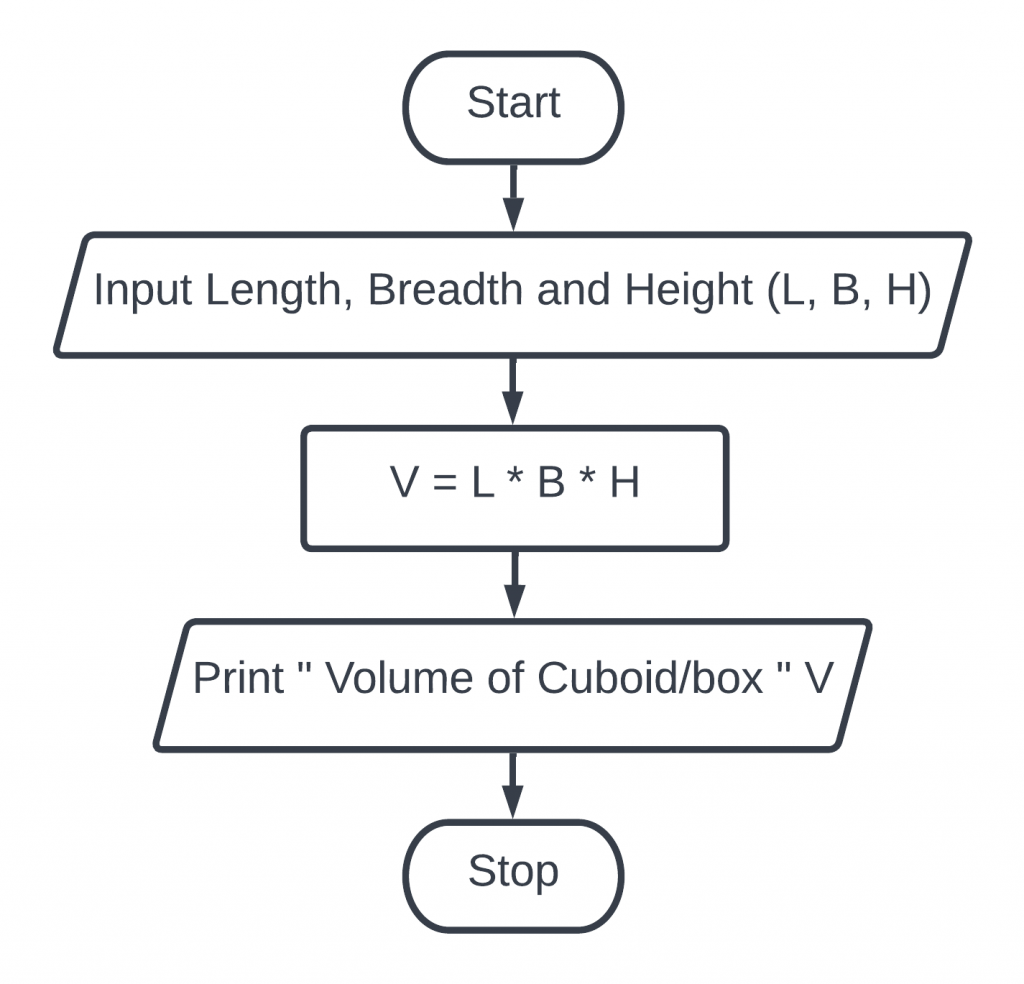
37. Create a flowchart and an algorithm to ask value in meter and convert it in inch.
Algorithm:
Step1: Start
Step 2:Input the length in Meter (M)
Step 3: I = M * 39.37
Step 4: Print “ Value In Inch “ I
Step 5: Stop/End
Flowchart:
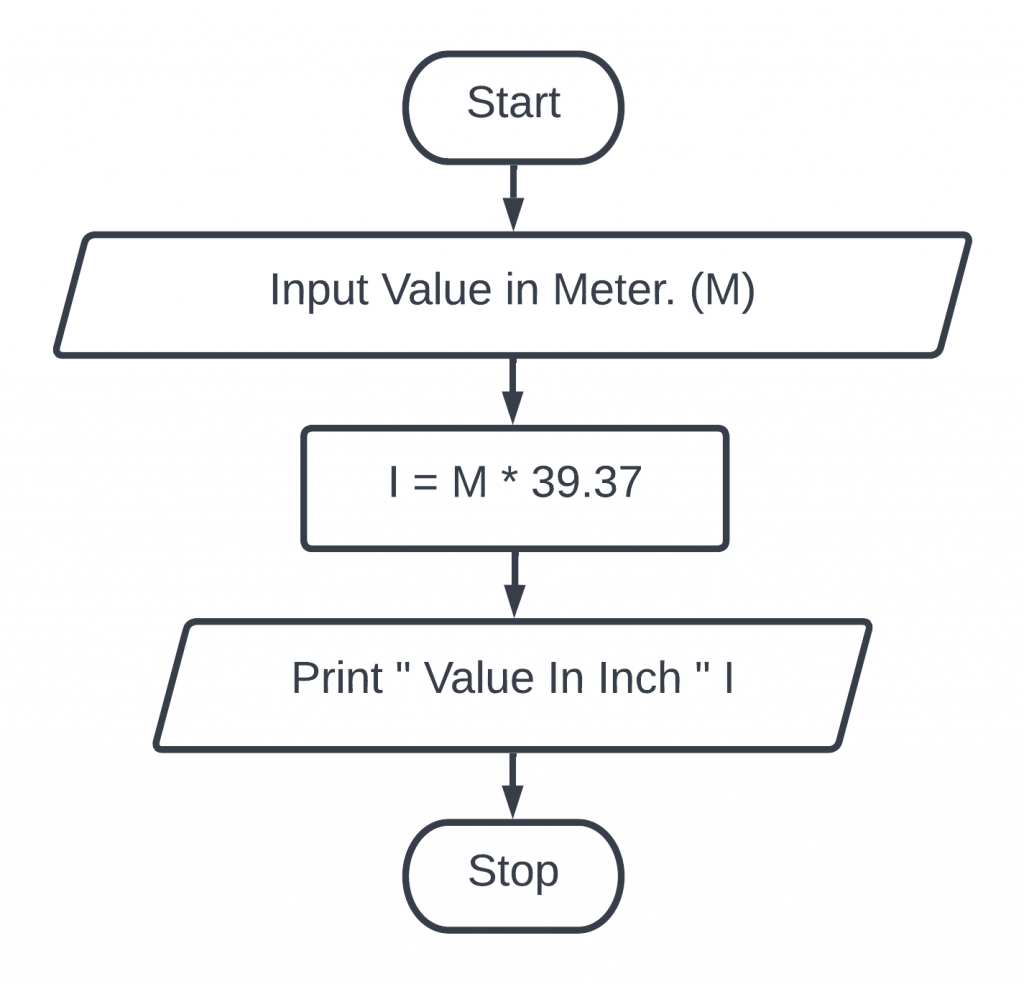
38. Create a flowchart and an algorithm to display total surface area and volume of hemisphere.
Algorithm:
Step 1: Start
Step 2: input Radius. ( R )
Step 3: A = 3 * 3.14 * R ^ 2
Step 4: V = ( 2 / 3 ) * 3.14 * R ^ 3
Step 5: Print “ Total Surface Area OF Hemisphere “ A
Step 6: Print “ Volume of Hemisphere “ V
Step 7: Stop/End
Flowchart:
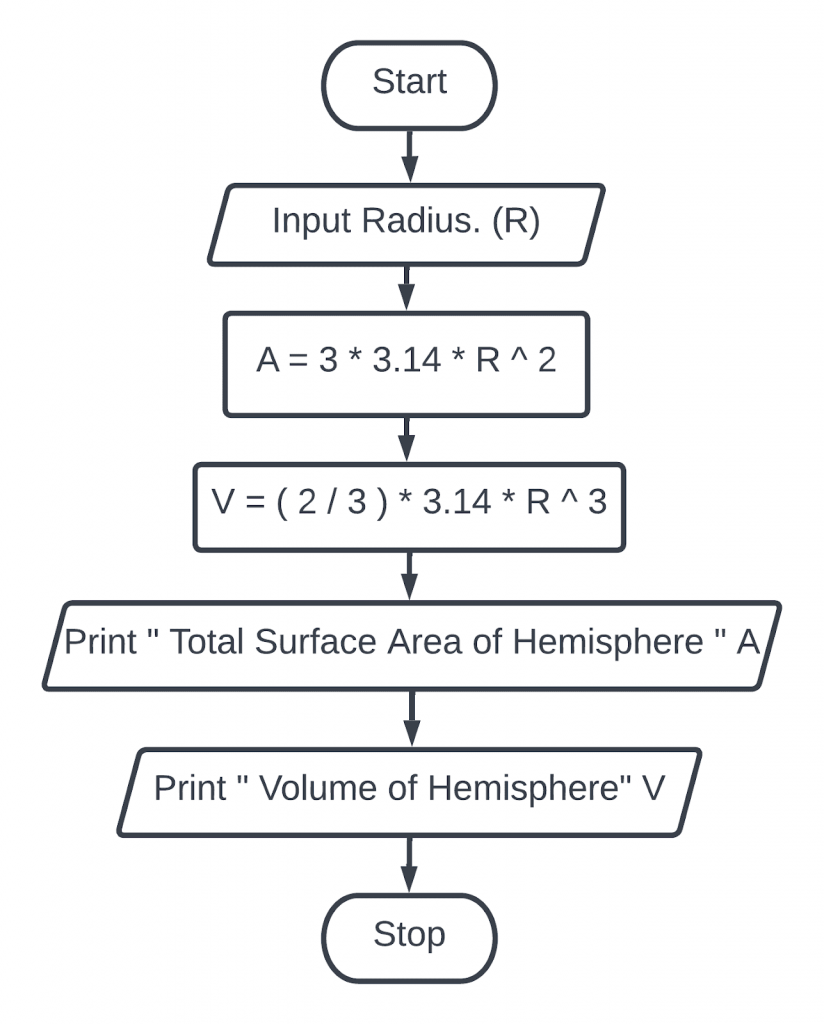
39. Create a flowchart and an algorithm to display area and perimeter of square.
Algorithm:
Step 1: Start
Step 2: Input Length. (L)
Step 3: A = L ^ 2
Step 4: P = 4 * L
Step 5: Print “ Area of Square “ A
Step 6: Print “ Perimeter of Square “ P
Step 6: Stop/End
Flowchart:
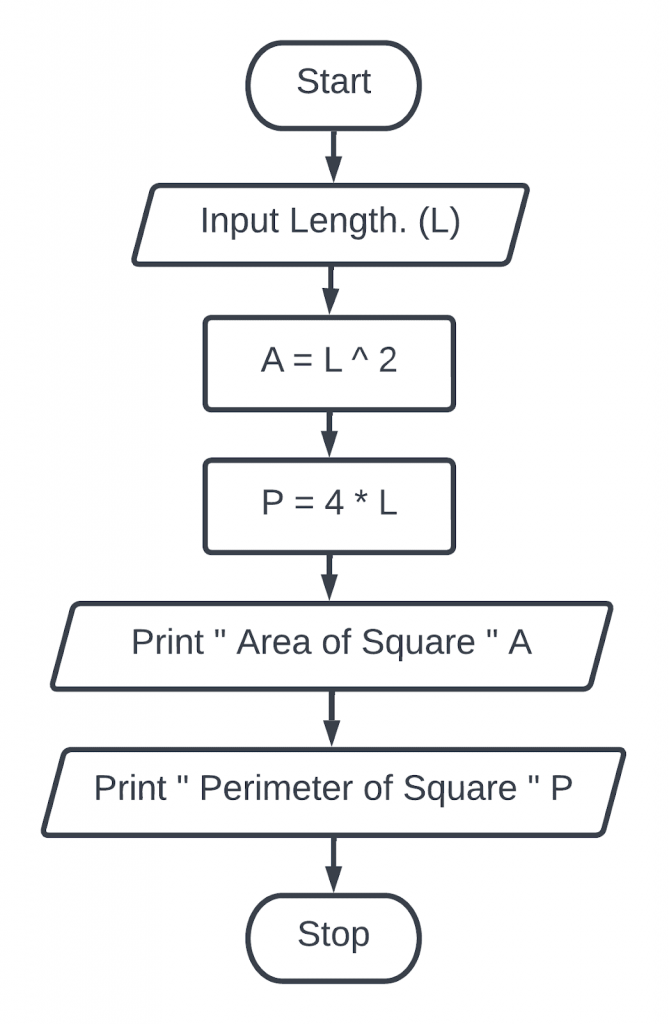
40. Create a flowchart and an algorithm to display cost of painting the four walls of a room.
Algorithm:
Step 1: Start
Step 2: Enter length, breadth, height and cost (l, b, h, c)
Step 3: A = 2 * h * (l + b)
Step 4: T = c * A
Step 4: Print Cost is :T
Step 5: Stop/End
Flowchart:
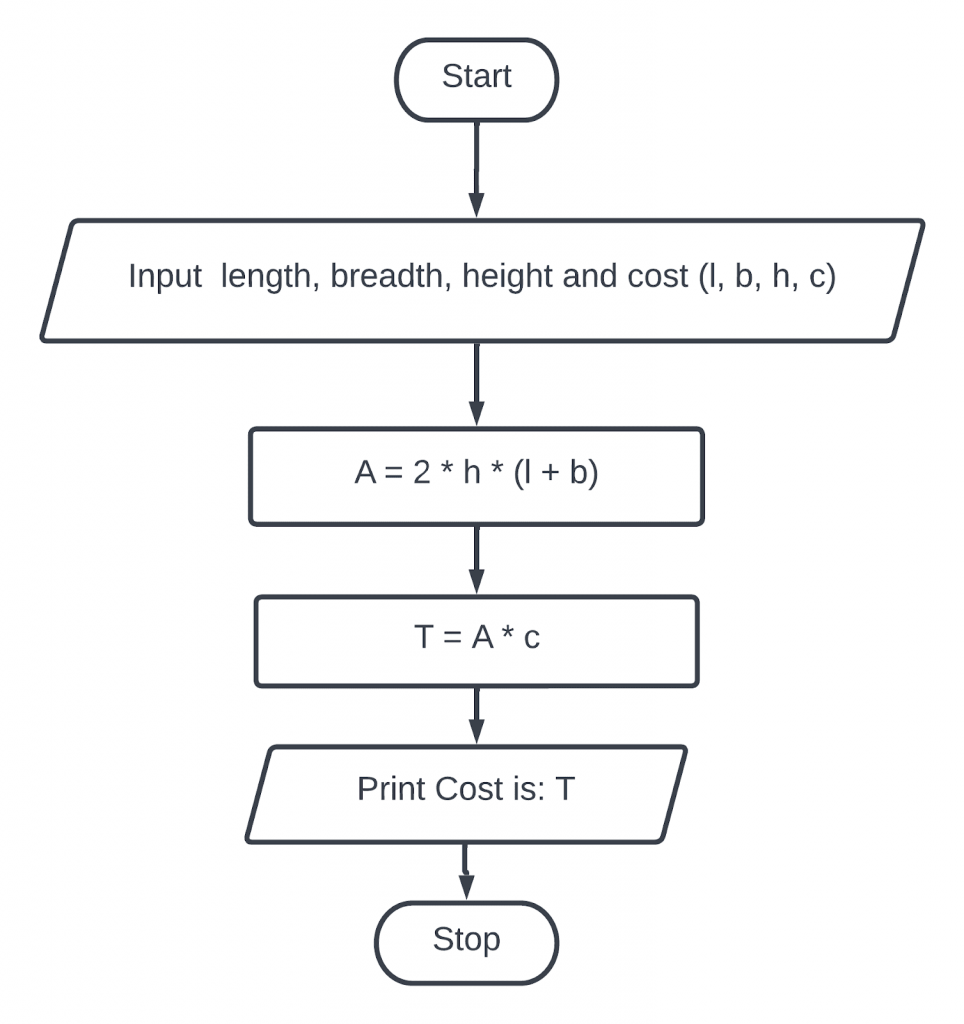