1. Create a flowchart and an algorithm to display the volume of the sphere .
Algorithm:
Step 1: Start
Step 2: Input Radius Of Sphere ( R )
Step 3: V = 4 / 3 * 3.14 * R ^ 3
Step 4: Print “Volume Of Sphere” V
Step 5: Stop/End
Flowchart:
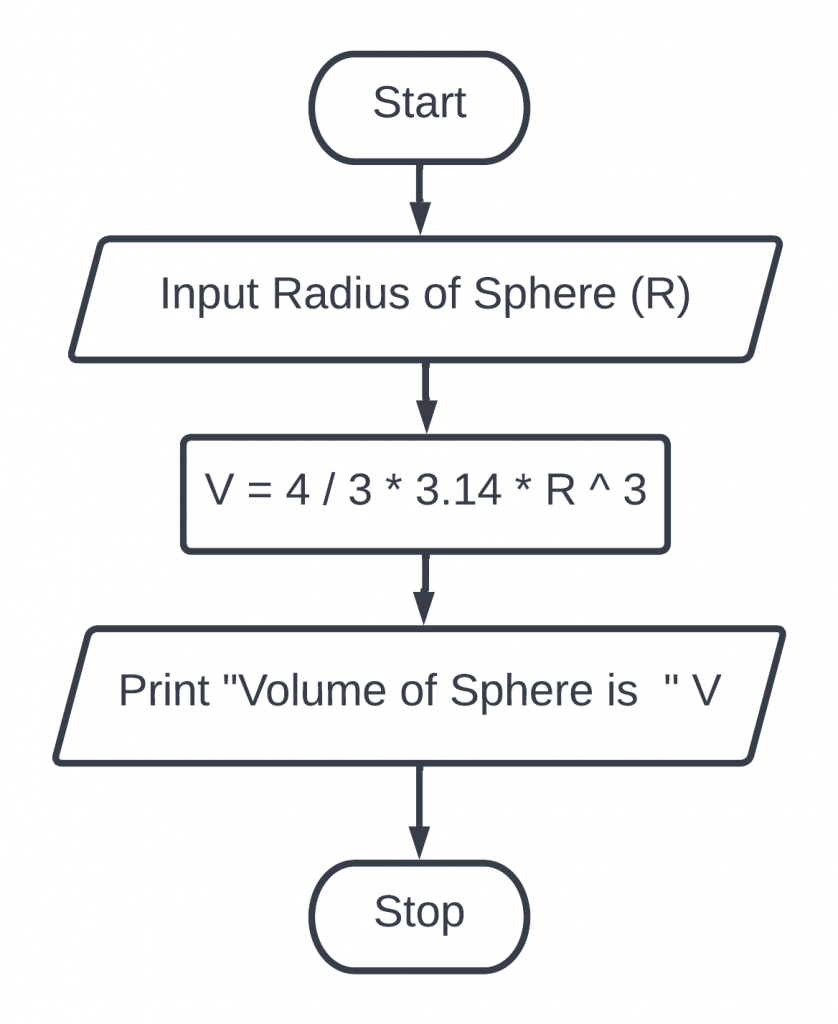
2. Create a flowchart and an algorithm to display an area of 4 walls.
Algorithm:
Step 1: Start
Step 2: Input length, breadth and height (l, b, h)
Step 3: A = 2 * h * ( l + b )
Step 4: Display A
Step 5: Stop/End
Flowchart:
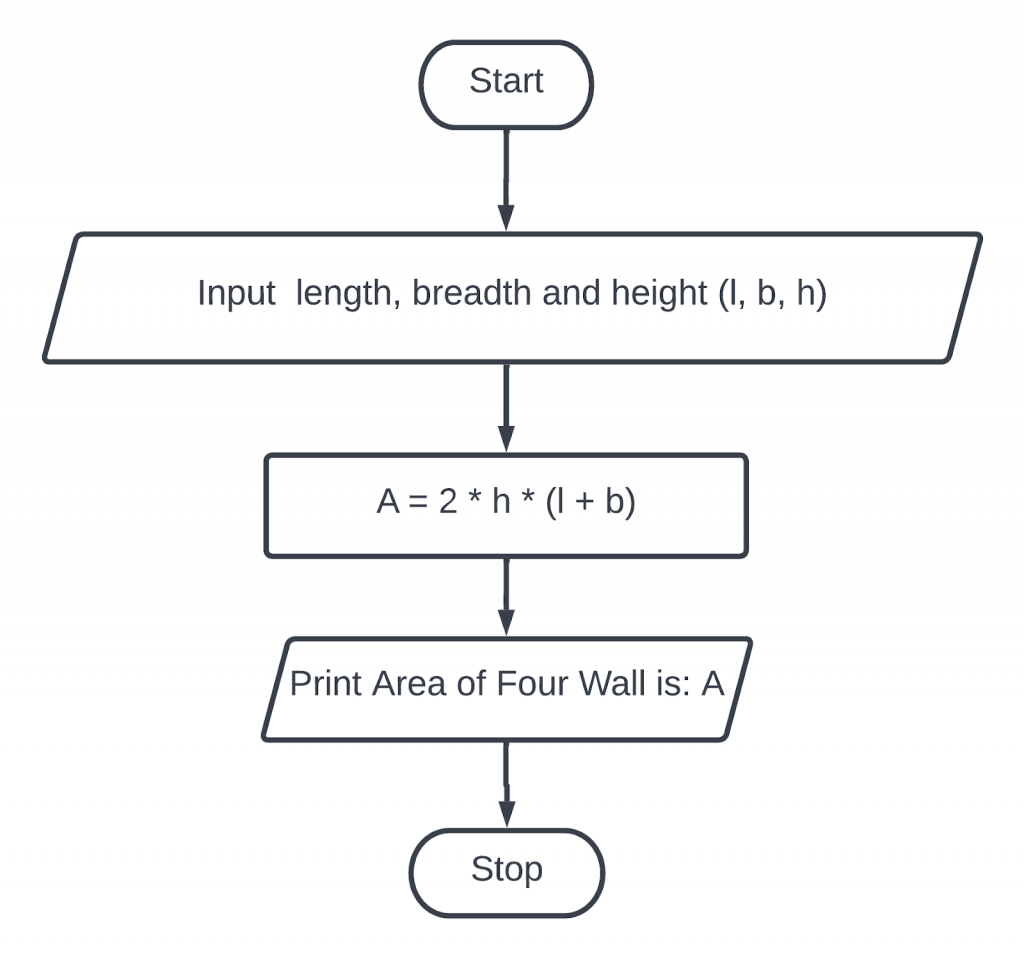
3.Create a flowchart and an algorithm to display the total surface area and volume of the cuboid
Algorithm:
Step 1: Start
Step 2: Input length, breadth and height of cuboid (l, b, h)
Step 3: A = 2 * ( l * b + b * h + h * l)
Step 4: V = l * b * h
Step 5: Print ”Total Surface Area Of Cuboid is” A
Step 6: Print “Volume Of Cuboid is ” V
Step 7: Stop/End
Flowchart:
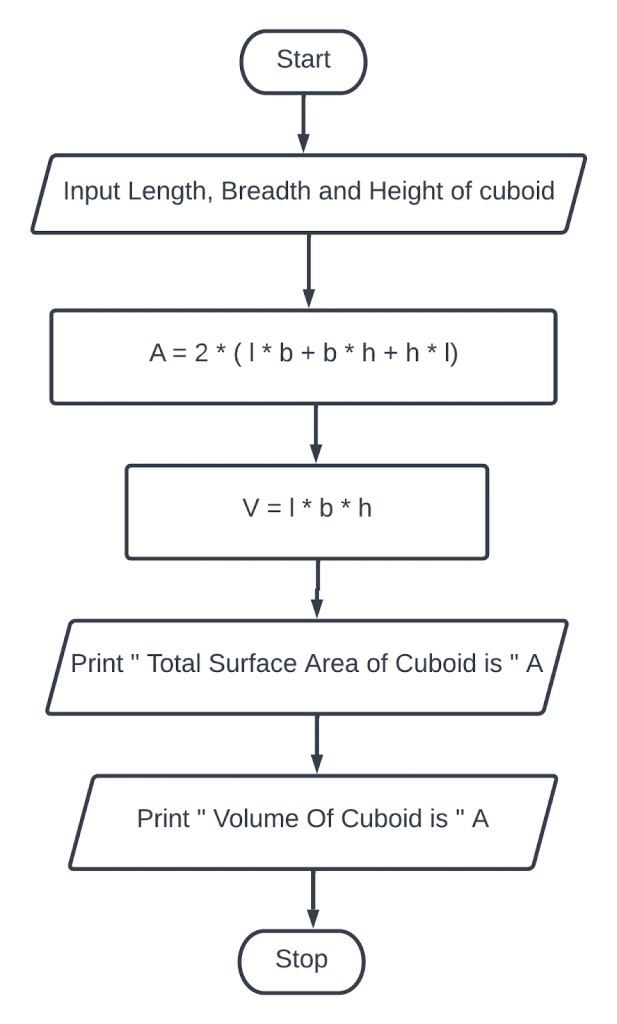
4. Create a flowchart and an algorithm to display the total surface area of cube.
Algorithm:
Step 1: Start
Step 2: Input Side of Cube(L)
Step 3: T = 6 * L ^ 2
Step 4: Print “ Total Surface Area of Cube” T
Step 5: Stop/End
Flowchart:
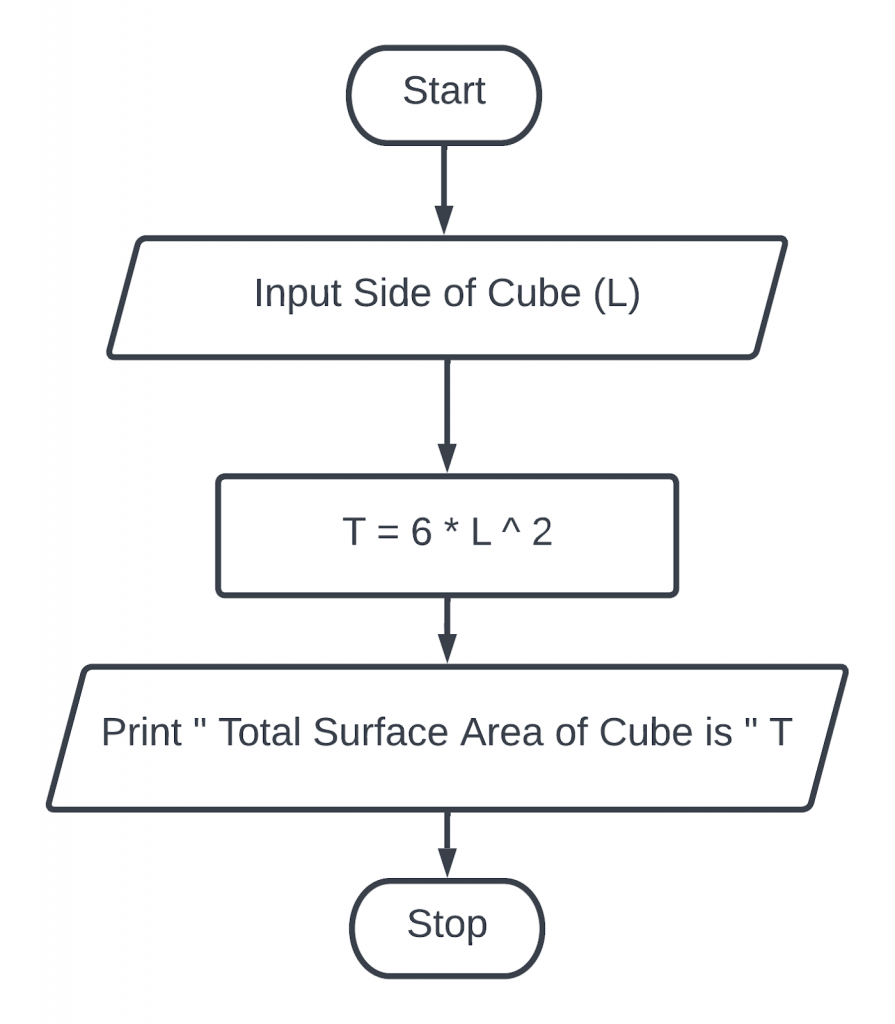
5. Create a flowchart and an algorithm to display Area of Rectangle.
Algorithm:
Step 1: Start
Step 2: Input length and breadth of rectangle (L ,B)
Step 3: A = L * B
Step 4: Print “ Area Of Rectangle ” A
Step 5: Stop/End
Flowchart:
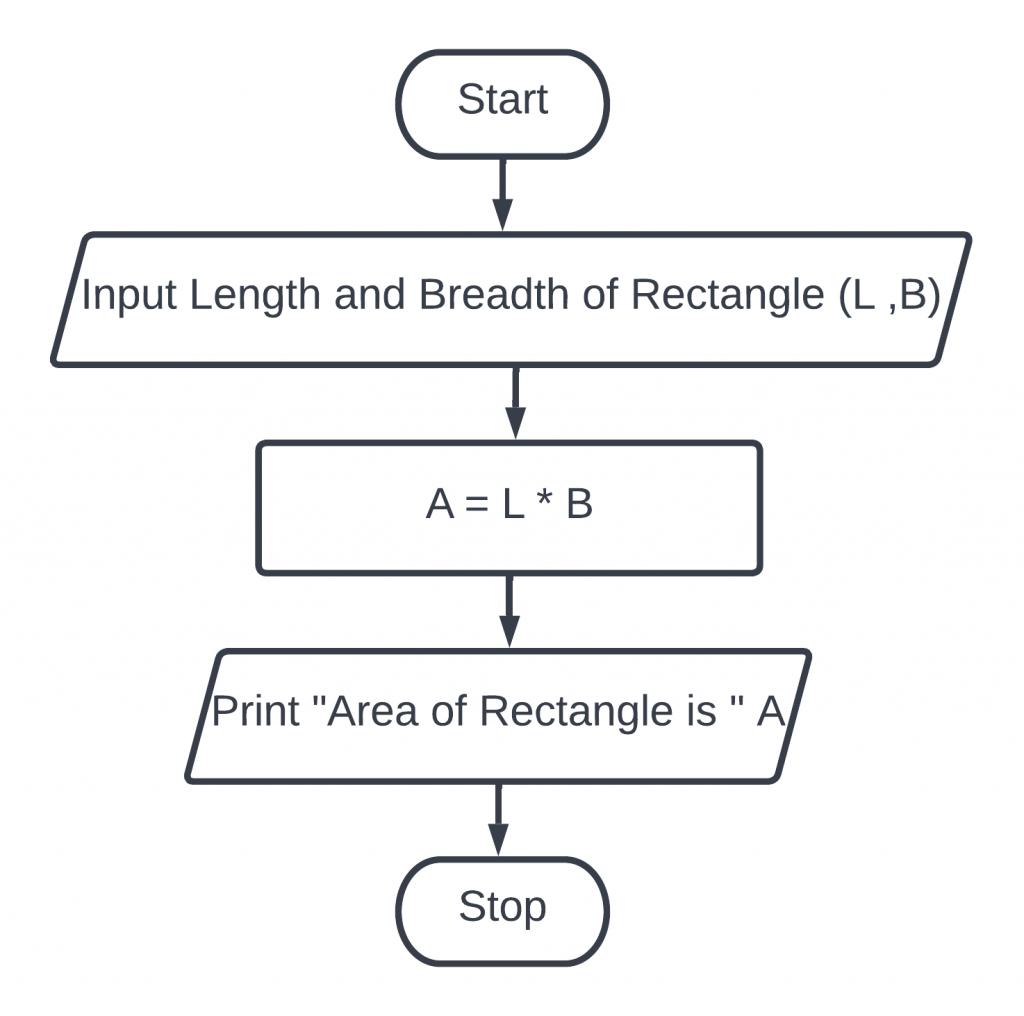
6. Create a flowchart and an algorithm to display Circumference of Circle.
Algorithm:
Step 1: Start
Step 2: Input Radius (R)
Step 3: C = 2 * 3.14 * R
Step 4: Print “ Circumference of Circle ” C
Step 5: Stop/End
Flowchart:
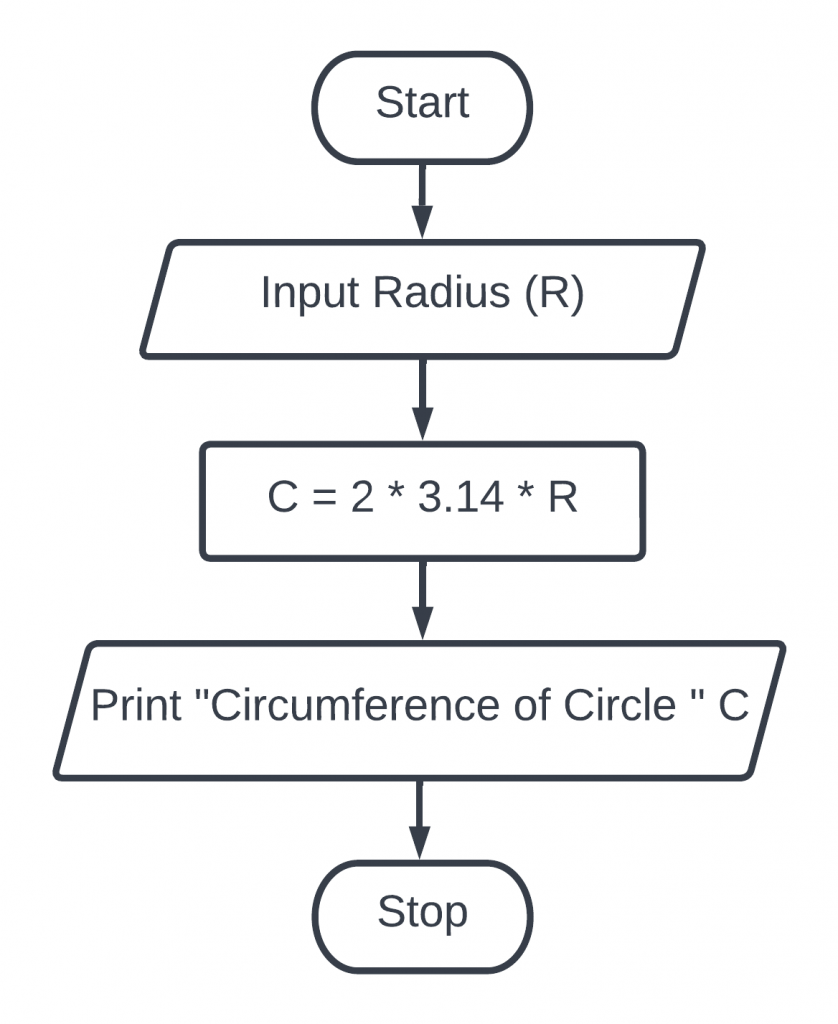
7. Create a flowchart and an algorithm to display area of triangle when three sides are given.
Algorithm:
Step 1: Start
Step 2: Input Three Sides of Triangle (a, b, c)
Step 3: s = a + b + c / 2
Step 4: AR = (s * (s – a) *(s – b)*(s-c)) ^ 1/ 2
Step 5: Print Area of Triangle is AR
Step 6: Stop/End
Flowchart:
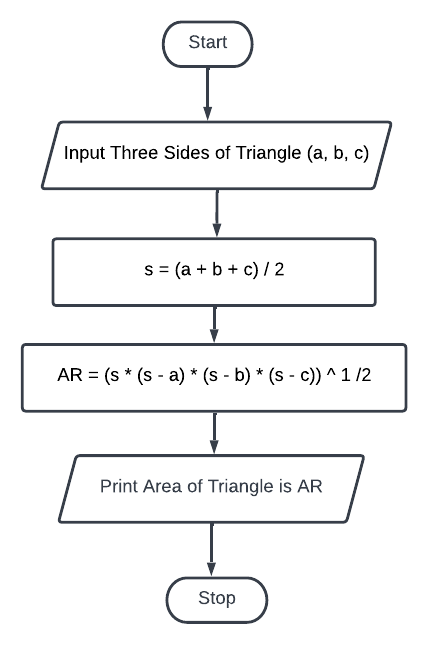
8. Create a flowchart and an algorithm to display Area and Circumference of Circle
Algorithm:
Step 1: Start
Step 2: Input Radius ( R )
Step 3: 3.14 * R ^ 2
Step 4: C = 2 * 3.14 * R
Step 5: Print “ Area of Circle ” A
Step 6: Print “ Circumference of Circle ” C
Step 7: Stop/End
Flowchart:
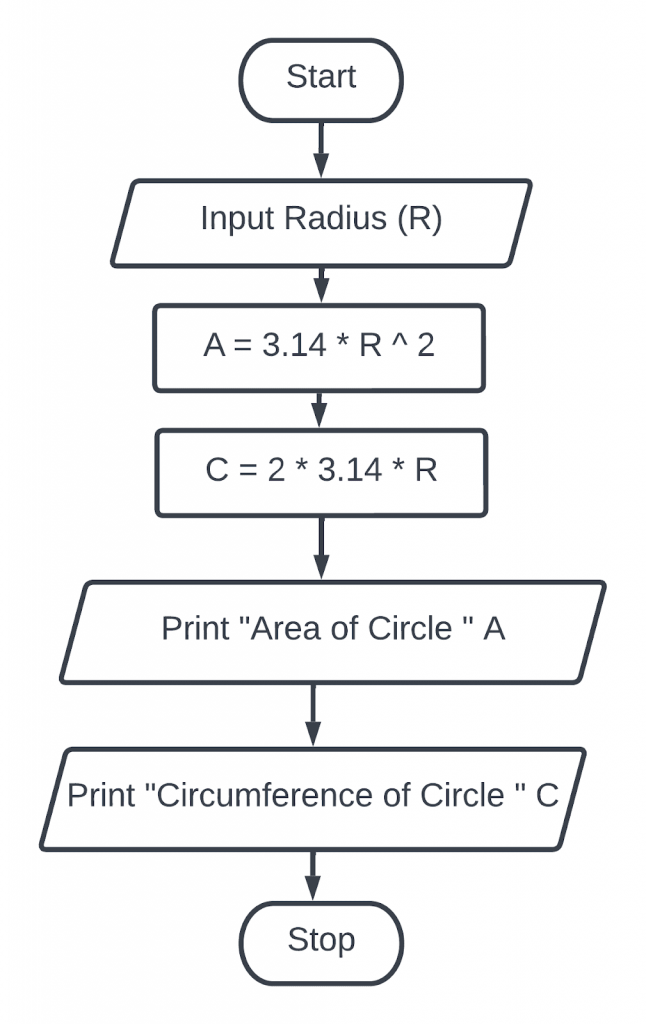
9. Create a flowchart and an algorithm to ask distance in kilometer and convert into miles.
Algorithm:
Step 1: Start
Step 2: Input distance in km (K)
Step 3: M = K * 0.62
Step 4: Print “ Distance in Miles” M
Step 5: Stop/End
Flowchart:
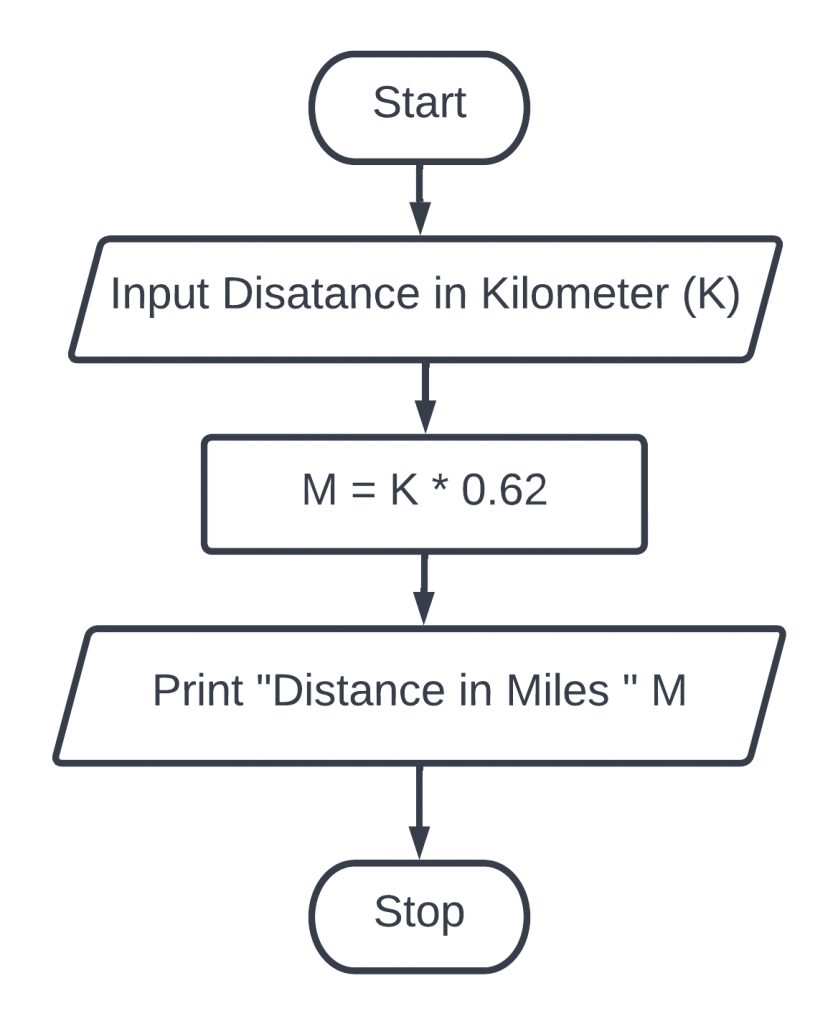
10. Create a flowchart and an algorithm to input distance in meter and convert into kilometer.
Algorithm:
Step 1: Start
Step 2: Input distance in meter(m)
Step 3: k = m / 1000
Step 4: Print Kilometer: k
Step 5: Stop/End
Flowchart:
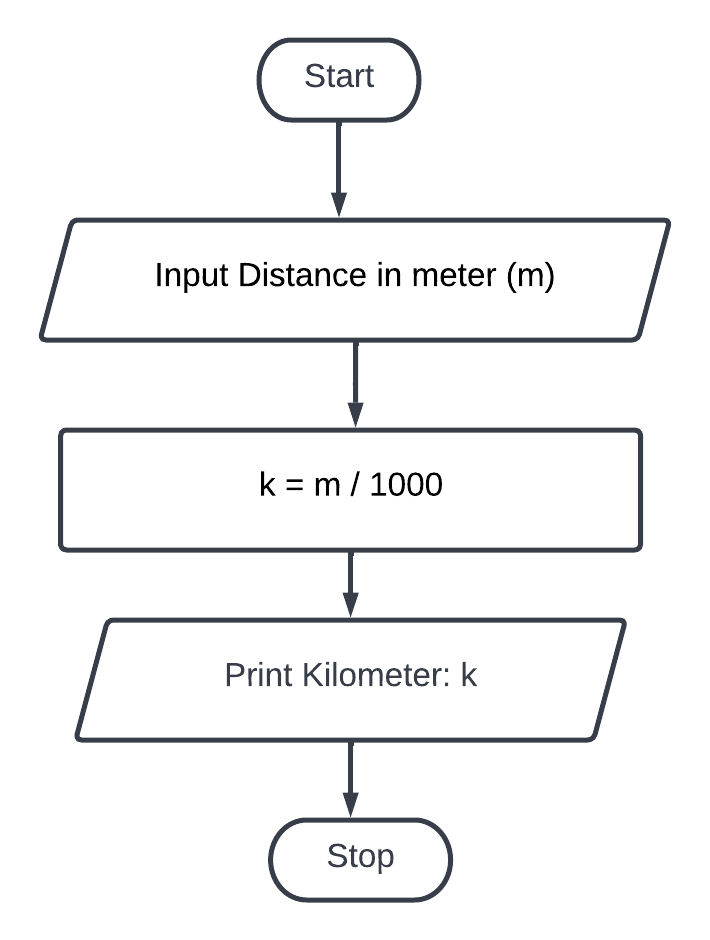